How to Set Worksheets in VBA
- Activating a Worksheet in VBA
- Setting a Worksheet Variable
- Looping Through Worksheets
- Referencing Worksheets by Index
- Conclusion
- FAQ
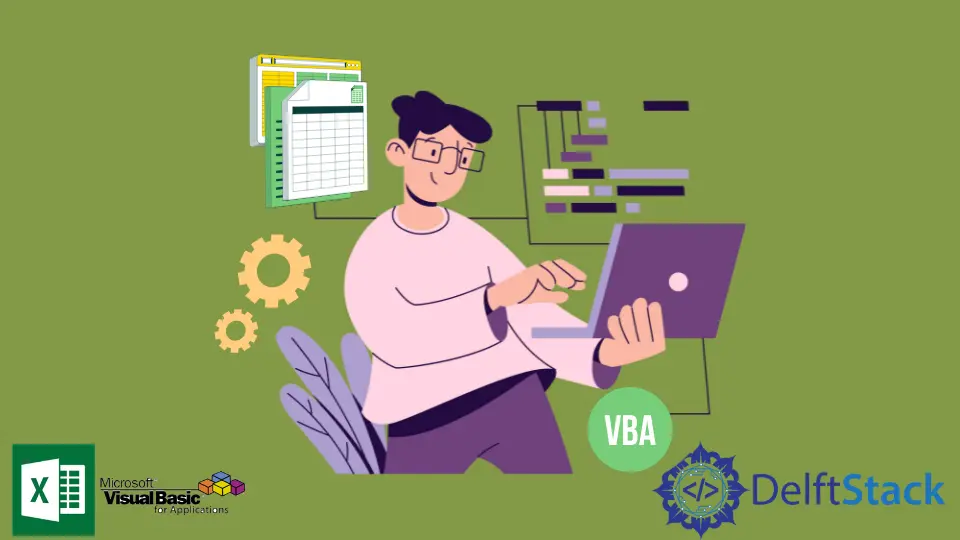
When working with Excel, managing worksheets efficiently can save you a lot of time and effort. Visual Basic for Applications (VBA) is a powerful tool that allows you to automate tasks and manipulate Excel worksheets with ease.
In this tutorial, we will dive into the various methods of setting worksheets in VBA. Whether you’re looking to activate a specific worksheet, reference worksheets dynamically, or even loop through multiple sheets, this guide has you covered. By the end, you will have a solid understanding of how to work with worksheets in VBA, making your Excel tasks more streamlined and efficient.
Activating a Worksheet in VBA
One of the most common tasks in VBA is activating a specific worksheet. This is crucial when you want to perform actions on a particular sheet. The Activate
method is straightforward and effective for this purpose.
Here’s how you can activate a worksheet using VBA:
Sub ActivateWorksheet()
Sheets("Sheet1").Activate
End Sub
When you run this code, it will bring “Sheet1” to the forefront of your Excel workbook. You can replace “Sheet1” with the name of any worksheet you wish to activate. This method is particularly useful when you want to ensure that your code interacts with the correct sheet, especially in workbooks with multiple sheets.
Output:
"Sheet1" is now activated.
Activating a worksheet is often the first step in a series of operations you may want to perform. Once activated, you can easily read or write data, format cells, or even create charts. However, it’s important to note that using Activate
can slow down your code if overused in larger projects. Instead, consider directly referencing the worksheet when performing operations.
Setting a Worksheet Variable
Another efficient way to work with worksheets in VBA is by setting a worksheet variable. This method allows you to reference the worksheet directly without repeatedly using the Sheets
or Worksheets
method. It makes your code cleaner and more efficient.
Here’s how to set a worksheet variable:
Sub SetWorksheetVariable()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
ws.Range("A1").Value = "Hello, World!"
End Sub
In this example, we declare a variable ws
of type Worksheet
and set it to reference “Sheet1”. This allows us to interact with “Sheet1” directly through the ws
variable. The code then assigns the value “Hello, World!” to cell A1 of “Sheet1”.
Output:
Cell A1 in "Sheet1" now contains "Hello, World!".
Using worksheet variables not only enhances readability but also improves performance, especially in larger macros. You can perform multiple operations on the ws
variable without needing to repeatedly specify the sheet name, which can reduce the risk of errors in your code.
Looping Through Worksheets
Sometimes, you may need to perform the same action across multiple worksheets. In such cases, looping through worksheets can be extremely useful. The For Each
loop allows you to iterate through each worksheet in your workbook efficiently.
Here’s an example of how to loop through all worksheets:
Sub LoopThroughWorksheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Cells(1, 1).Value = "Data in " & ws.Name
Next ws
End Sub
In this code, we declare a Worksheet
variable ws
and use the For Each
loop to iterate through every worksheet in the current workbook. For each worksheet, we set the value of cell A1 to indicate the name of the worksheet.
Output:
Cell A1 in each worksheet now contains "Data in [WorksheetName]".
This method is particularly handy when you need to apply the same formatting or data entry across multiple sheets. It saves time and ensures consistency across your workbook. Just remember to be careful with the actions you perform within the loop, as changes will affect all worksheets.
Referencing Worksheets by Index
In some cases, you may want to reference worksheets by their index rather than by name. This can be particularly useful when the names of the sheets may change or when you don’t know the names in advance. The Worksheets
collection allows you to access sheets using their index number.
Here’s how to reference a worksheet by its index:
Sub ReferenceWorksheetByIndex()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets(1)
ws.Range("B1").Value = "First Worksheet"
End Sub
This code sets the variable ws
to the first worksheet in the workbook. It then assigns the text “First Worksheet” to cell B1 of that sheet.
Output:
Cell B1 in the first worksheet now contains "First Worksheet".
Referencing worksheets by index is particularly useful in cases where the sheet names are dynamic or not known at design time. However, keep in mind that using index numbers can lead to errors if sheets are added or removed, so it’s best used in stable environments.
Conclusion
Setting worksheets in VBA is a fundamental skill that can greatly enhance your productivity when working with Excel. Whether you’re activating sheets, setting variables, looping through multiple worksheets, or referencing them by index, understanding these methods will empower you to automate tasks efficiently. With practice, you’ll find that VBA can significantly streamline your workflow, allowing you to focus on more important aspects of your work.
FAQ
-
How do I activate a worksheet in VBA?
You can activate a worksheet using theActivate
method, like this:Sheets("SheetName").Activate
. -
What is a worksheet variable in VBA?
A worksheet variable is a variable that holds a reference to a specific worksheet, allowing you to interact with it directly without repeatedly specifying its name. -
How do I loop through all worksheets in a workbook?
You can use aFor Each
loop to iterate through all worksheets:For Each ws In ThisWorkbook.Worksheets
. -
Can I reference a worksheet by its index in VBA?
Yes, you can reference a worksheet by its index usingWorksheets(indexNumber)
. -
What are the advantages of using worksheet variables?
Worksheet variables improve code readability and performance, allowing for cleaner and more efficient code.