How to Use Quotes in Strings in VBA
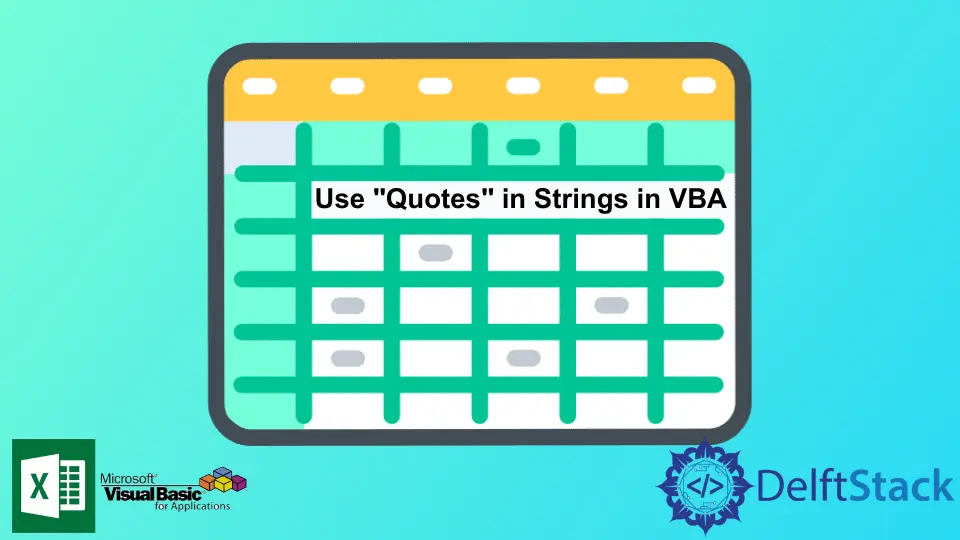
We will introduce how to use double quotes in strings in VBA with some examples.
Use Quotes in Strings in VBA
While working with VBA, we have always wondered what to do if we want to include double quotes in a string and use them in our functions without any problems. There are multiple ways to use the double quotes inside strings without getting into any errors and problems.
We will discuss these methods one by one with examples.
Use Extra Double Quotes to Use Quotes in Strings in VBA
The first example is by using extra double quotes. If we use two extra double quotes, it will include only one double quote inside the string.
Let’s go through an example and use this method as shown below.
# VBA
Sub addDoubleQuotes()
Value = "This is a string including ""doublequotes"""
Selection.Value = Value
End Sub
Output:
As we can see from the example, we can easily add one double quote inside a string by adding two double quotes.
Use the Character Code for Double Quotes to Use Quotes in Strings in VBA
Let’s now go through another example in which we will use a character code to add double quotes inside our string, as shown below.
# VBA
Sub addDoubleQuotes()
Value = "This is a string including " & Chr(34) & " doublequotes " & Chr(34)
Selection.Value = Value
End Sub
Output:
As we can see from the example, by using the character code for double quotes, which is Chr(34)
, we can easily add double quotes inside strings in VBA.