How to Open PDF in VBA
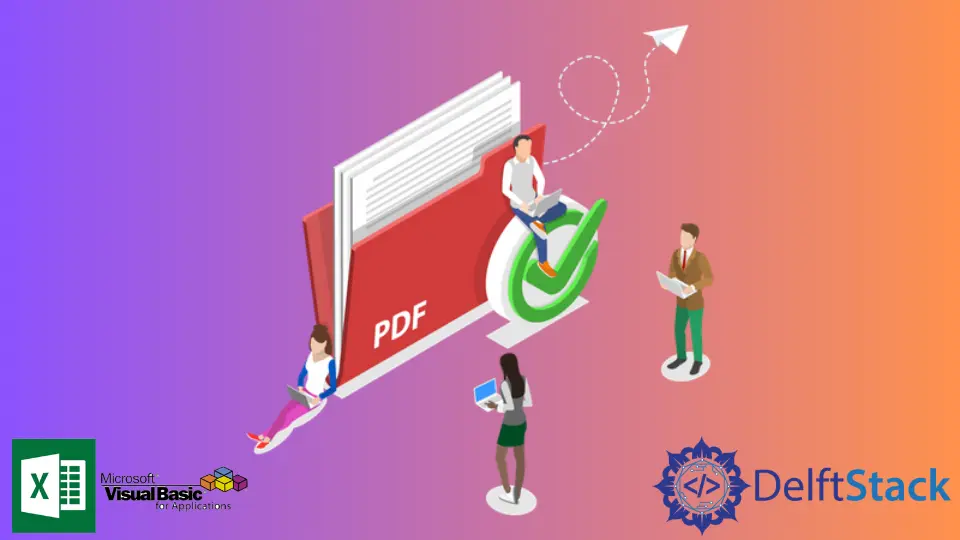
In this article, we will learn how we can open a PDF file using VBA with the help of an example.
Open PDF in VBA
VBA’s complete form is Visual Basic for Applications. It is also a programming language that Microsoft develops, and they still own this language.
With the help of VBA, we can automate many functions in Excel files. We can use VBA coding to create Macros that can perform specific functions.
With the help of VBA, we can automate specific tasks. We can even use the VBA to extract PDF files data and put them inside Excel.
We can even use the VBA to convert the PDF files into Excel files.
While working on VBA, there may be some situations in which we may want to open a PDF file to check something, or some function needs to open a PDF file. For this purpose, there are some solutions available in VBA.
If the purpose of the function is to open the PDF file, we can use the FollowHyperLink
and give the path of the PDF file that we want to open.
As shown below, let’s go through an example and try to use this method in our example.
Sub openPDF()
ActiveWorkbook.FollowHyperLink "E:\new.pdf"
End Sub
This method will open the PDF file in the default PDF viewer on our system. We can use this method to open any PDF file or any file in our system by using VBA.