How to Return Array From Function in VBA
- Understanding Arrays in VBA
- Returning an Array from a Function
- Utilizing the Returned Array
- Conclusion
- FAQ
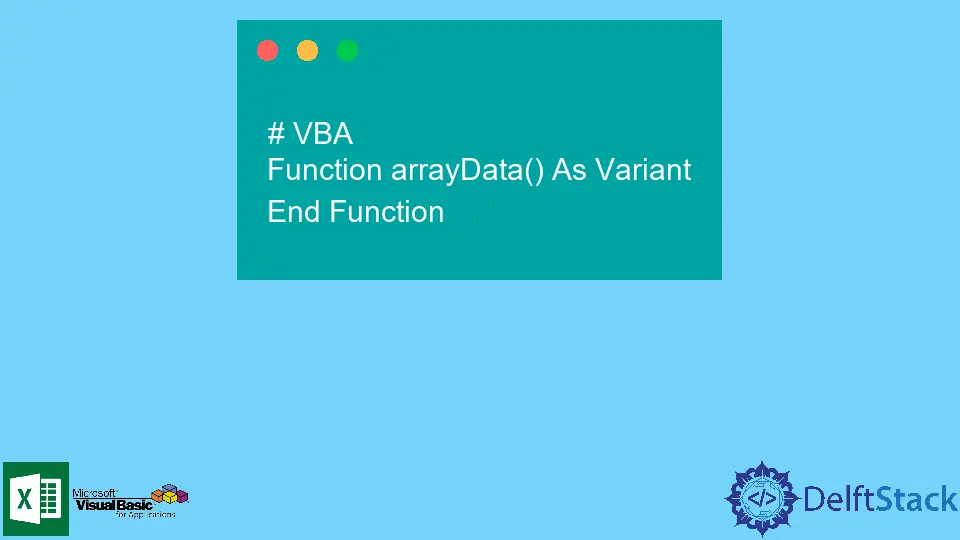
Returning an array from a function in VBA can significantly enhance your programming efficiency. Whether you are working on data manipulation, calculations, or simply organizing data, understanding how to return arrays will open new doors for your VBA projects.
In this article, we will walk you through the steps required to return an array from a function in VBA. You’ll learn the syntax, see practical examples, and discover how to effectively utilize arrays within your functions. So, let’s dive into the world of VBA and explore the power of arrays!
Understanding Arrays in VBA
Before we jump into the code, it’s essential to clarify what an array is. In VBA, an array is a collection of variables that are accessed using a single name and an index number. Arrays can hold multiple values of the same type, making them a powerful tool for managing data efficiently.
Declaring an Array
To return an array from a function, you first need to declare the array properly. You can declare a dynamic array, which allows you to define the size of the array at runtime. Here’s a simple example of how to declare a dynamic array in a function.
Function CreateArray() As Variant
Dim myArray() As Variant
ReDim myArray(1 To 5) As Variant
myArray(1) = "Apple"
myArray(2) = "Banana"
myArray(3) = "Cherry"
myArray(4) = "Date"
myArray(5) = "Elderberry"
CreateArray = myArray
End Function
This code defines a function called CreateArray
. Inside the function, we declare a dynamic array named myArray
and use ReDim
to set its size. We then populate the array with fruit names and return it.
Output:
Apple
Banana
Cherry
Date
Elderberry
When you call CreateArray
, it returns an array containing five types of fruits. This is a straightforward way to return an array from a function in VBA.
Returning an Array from a Function
Now that we have a basic understanding of arrays, let’s explore how to return an array from a function effectively. This involves defining the function’s return type and properly assigning the array to that return type.
Example of Returning an Array
Here’s a more elaborate example that demonstrates how to return a two-dimensional array from a function. This can be particularly useful if you are dealing with tabular data.
Function Create2DArray() As Variant
Dim myArray(1 To 3, 1 To 2) As Variant
myArray(1, 1) = "Name"
myArray(1, 2) = "Age"
myArray(2, 1) = "John"
myArray(2, 2) = 30
myArray(3, 1) = "Jane"
myArray(3, 2) = 25
Create2DArray = myArray
End Function
In this example, we define a function called Create2DArray
, which returns a two-dimensional array. We initialize the array to hold names and ages, and then we return this array.
Output:
Name Age
John 30
Jane 25
This function can be called from anywhere in your VBA project, and it will return a structured array containing both names and their corresponding ages.
Utilizing the Returned Array
Once you have a function that returns an array, the next step is to utilize that array effectively. You can loop through the array to process its contents. Here’s how you can do that.
Example of Looping Through the Returned Array
Sub DisplayArray()
Dim fruits As Variant
Dim i As Integer
fruits = CreateArray()
For i = LBound(fruits) To UBound(fruits)
Debug.Print fruits(i)
Next i
End Sub
In this code snippet, we declare a subroutine called DisplayArray
. We call the CreateArray
function to get the array of fruits and then use a For
loop to print each fruit to the Immediate Window.
Output:
Apple
Banana
Cherry
Date
Elderberry
This approach allows you to leverage the power of arrays returned from functions, making your code cleaner and more efficient.
Conclusion
Returning an array from a function in VBA can greatly enhance your programming capabilities. By mastering this technique, you can streamline your data management and processing tasks. We explored how to declare arrays, return them from functions, and utilize them effectively. Whether you’re creating simple lists or complex data structures, understanding how to work with arrays will empower your VBA projects. Embrace the power of arrays, and watch your coding skills soar!
FAQ
-
How do I declare an array in VBA?
You can declare an array in VBA using the Dim statement, and you can use ReDim to define its size dynamically. -
Can I return a multi-dimensional array from a function in VBA?
Yes, you can return multi-dimensional arrays from functions in VBA by declaring the array with multiple dimensions and returning it as a Variant.
-
What is the difference between a static and dynamic array in VBA?
A static array has a fixed size defined at declaration, while a dynamic array can be resized at runtime using ReDim. -
How do I loop through an array in VBA?
You can loop through an array using a For loop, utilizing the LBound and UBound functions to determine the array’s boundaries. -
Can I return an array of different data types from a function in VBA?
Yes, you can return an array containing different data types by declaring the array as a Variant.