How to Call Sub in VBA
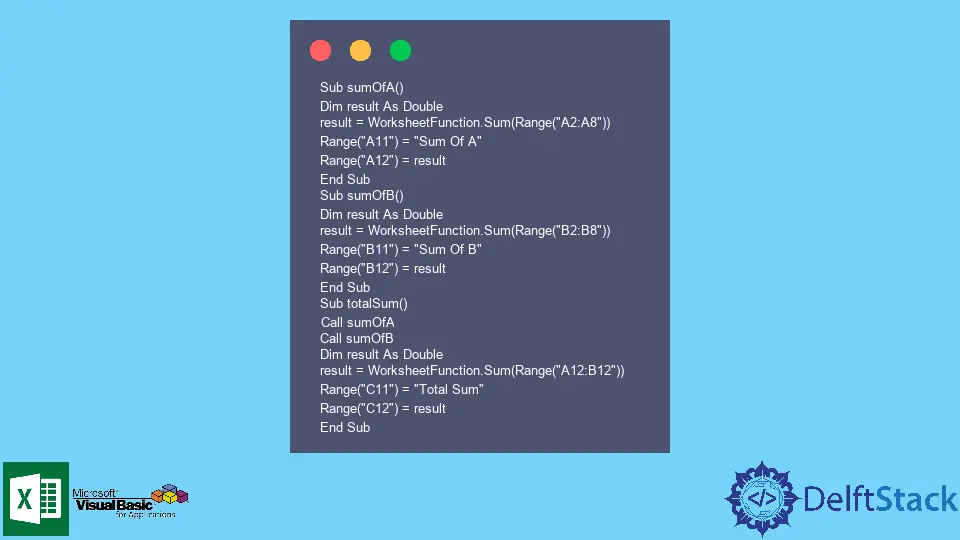
We will introduce how to call a sub in another sub with an example in VBA.
Call Sub in VBA
While working on multiple sub-procedures, we may encounter a situation where we may need to call multiple sub-procedures for the same function.
Some functions require a huge amount of code to be written, and to make this code easily understandable; we need to make them in multiple parts. And we need to call these parts in a single sub to make sure that the whole process works in a streamline.
This tutorial will teach us to call multiple subs in a single sub. Let’s go through an example in which we will get the sum of multiple columns and then get the sum of the multiple sums, as shown below.
Sub sumOfA()
Dim result As Double
result = WorksheetFunction.Sum(Range("A2:A8"))
Range("A11") = "Sum Of A"
Range("A12") = result
End Sub
Sub sumOfB()
Dim result As Double
result = WorksheetFunction.Sum(Range("B2:B8"))
Range("B11") = "Sum Of B"
Range("B12") = result
End Sub
Sub totalSum()
Call sumOfA
Call sumOfB
Dim result As Double
result = WorksheetFunction.Sum(Range("A12:B12"))
Range("C11") = "Total Sum"
Range("C12") = result
End Sub
Output:
As we can see from the above example, we wanted to get the sum of multiple columns, and after that, we wanted to get the sum of the sums we got. For this purpose, we broke down the code into three different subs and called the two subs in our last sub.
Breaking code into multiple functions is a good practice to make them simple and easy.