How to Utilize Comments on VBA
- What are Comments in VBA?
- Best Practices for Writing Comments in VBA
- Utilizing Comments for Debugging
- How to Manage Comments in VBA
- Conclusion
- FAQ
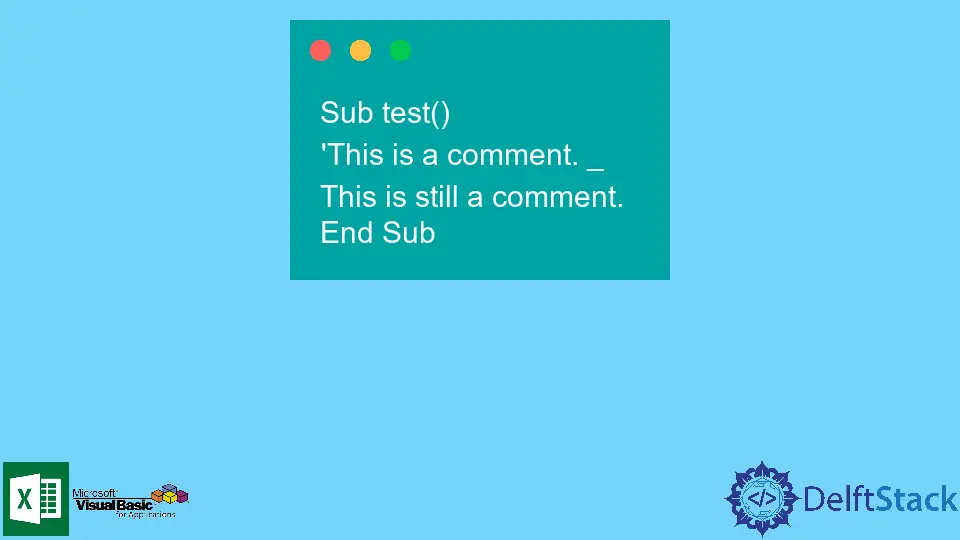
VBA, or Visual Basic for Applications, is a powerful tool for automating tasks in Microsoft Office applications. One of the most effective practices when working with VBA is the use of comments. Comments are crucial for making your code more understandable, both for yourself and for others who may read it later.
In this tutorial, we will explore how to create and manage comments in VBA, ensuring that your code is not only functional but also easy to follow. By the end, you’ll see how comments can enhance your coding experience and improve collaboration in projects. Let’s dive into the world of VBA comments!
What are Comments in VBA?
Comments in VBA are lines of text that are not executed as part of the code. They are used to explain what the code does, making it easier to understand. In VBA, you can add comments by using an apostrophe ('
). Everything following the apostrophe on that line is treated as a comment. This simple feature can drastically enhance the readability of your code.
Here’s a basic example:
Sub ExampleSub()
' This line declares a variable
Dim myVariable As Integer
myVariable = 10 ' Assign 10 to myVariable
End Sub
In the code above, the comments provide context for what each line does. The first comment explains that a variable is being declared, while the second comment clarifies the assignment operation. This can be especially helpful when revisiting your code after some time or when sharing it with colleagues.
Best Practices for Writing Comments in VBA
Writing effective comments is an art. Here are some best practices to keep in mind:
- Be Concise: Comments should be brief yet informative. Avoid lengthy explanations; instead, aim for clarity.
- Use Proper Grammar: Well-written comments enhance professionalism and make your code easier to read.
- Comment on Complex Logic: If you have a particularly complicated piece of code, take the time to explain it in detail.
- Update Comments: Ensure that comments are updated when the code changes. Outdated comments can confuse readers.
For instance, consider this more complex example:
Sub CalculateTotal()
' This subroutine calculates the total sales
Dim sales As Double
Dim tax As Double
Dim total As Double
sales = 100.0 ' Sales amount
tax = 0.05 ' Tax rate
' Calculate total including tax
total = sales + (sales * tax)
' Display total
MsgBox "Total sales including tax: " & total
End Sub
In this example, comments clarify the purpose of each variable and explain the calculations being performed. This approach makes it easier for anyone reviewing the code to grasp its functionality quickly.
Utilizing Comments for Debugging
Comments can also be a powerful tool for debugging. By commenting out specific lines of code, you can isolate issues without deleting code. This allows you to test various parts of your program without losing your original work.
Here’s how you can use comments for debugging:
Sub DebugExample()
Dim result As Integer
Dim value As Integer
value = 5
' Uncomment the next line to test with a different value
' value = 10
result = value * 2 ' Calculate double the value
MsgBox "The result is: " & result
End Sub
In this code, the line that changes the value of value
is commented out. By simply uncommenting it, you can test how the program behaves with a different input. This method is efficient and keeps your code intact while allowing for quick iterations.
Output:
The result is: 10
This approach not only saves time but also helps maintain the integrity of your original code, allowing for easy experimentation.
How to Manage Comments in VBA
Managing comments effectively in your VBA projects can streamline your workflow. Here are some strategies:
- Use Block Comments: For larger sections of code, consider using block comments. You can do this by preceding multiple lines with an apostrophe. Alternatively, you can use the
#If
directive for conditional compilation.
-
Commenting Style: Establish a consistent commenting style throughout your code. Whether you prefer single-line comments or block comments, consistency makes your code easier to navigate.
-
Documentation: For larger projects, consider creating a separate documentation file that explains the overall structure and purpose of your code. This can be linked within your comments.
Here’s an example demonstrating block comments:
Sub ExampleWithBlockComments()
' This subroutine does the following:
' 1. Initializes variables
' 2. Performs calculations
' 3. Displays results
Dim x As Integer, y As Integer, result As Integer
x = 10
y = 20
result = x + y ' Summing x and y
MsgBox "The result is: " & result
End Sub
In this example, the block comment at the beginning provides a summary of what the subroutine does. This is particularly useful for larger projects where understanding the overall function at a glance is crucial.
Output:
The result is: 30
By implementing these strategies, you can significantly enhance the clarity and maintainability of your VBA code.
Conclusion
Utilizing comments in VBA is an essential practice for anyone looking to write clear and maintainable code. By incorporating comments effectively, you can enhance your code’s readability, facilitate debugging, and improve collaboration with others. Remember to keep your comments concise, relevant, and up to date. With these tips in mind, you’ll be well on your way to mastering comments in VBA, making your coding experience more enjoyable and productive.
FAQ
-
What is the purpose of comments in VBA?
Comments in VBA are used to explain the code, making it easier to understand and maintain. -
How do you create a comment in VBA?
You can create a comment in VBA by using an apostrophe ('
) before the comment text. -
Can comments affect the performance of my VBA code?
No, comments do not affect the performance of your code as they are ignored during execution. -
Should I comment every line of code?
No, only comment on complex logic or when necessary to clarify the purpose of a section of code. -
How can I quickly comment out multiple lines of code in VBA?
You can comment out multiple lines by adding an apostrophe before each line or using the#If
directive for conditional compilation.