How to Round Up Numbers in VBA
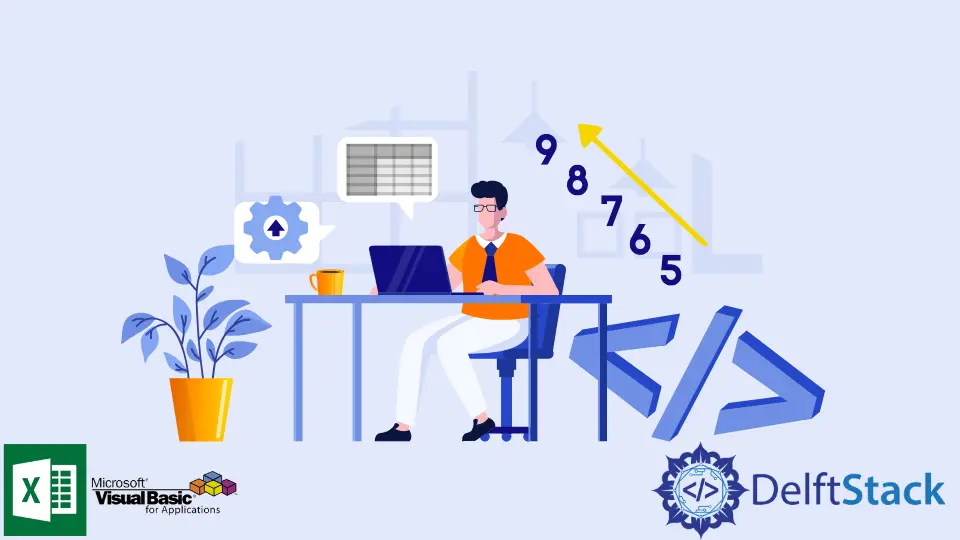
We will use examples to introduce the Round()
and RoundUp()
functions in VBA.
Use the Round()
or the RoundUp()
Functions in VBA
While working with excel sheets that contain numbers and calculations, we get results in fractions. Sometimes we have to convert these decimal numbers into whole numbers.
VBA provides three different functions that can be used to convert decimal numbers into whole numbers. We will discuss two functions in this article.
The Round()
round the decimal number to the nearest whole number. For example, we will store a decimal number and then use the Round()
function.
Example Code:
# VBA
Sub roundFunc()
Dim num As Double
num = 5.79
MsgBox Round(num)
End Sub
Output:
We can even mention the number of decimal places till we want to round off the decimal number. Let’s try to round off the same number up to the one decimal place.
Example Code:
# VBA
Sub roundFunc()
Dim num As Double
num = 5.79
MsgBox Round(num, 1)
End Sub
Output:
From the above result, we can easily round off the decimal number up to 1 decimal place. We can also use this function to round off the value from the cell in the excel sheet.
Let’s try round the number in the excel sheet using the range method.
Example Code:
# VBA
Sub roundFunc()
Range("A1").Value = Round(Range("A1").Value, 1)
End Sub
Output:
Let’s discuss another function that can round up the decimal number even if the number is near to the smaller whole number. There may be many situations where we must always round off the decimal number to the whole number greater than the decimal number.
Syntax:
# VBA
Application.WorksheetFunction.RoundUp(num, 1)
Let’s have an example and try to use the RoundUp()
function.
Example Code:
# VBA
Sub roundFunc()
Dim num As Double
num = 5.468
MsgBox Application.WorksheetFunction.RoundUp(num, 1)
End Sub
Output:
This way, we can round off the numbers to the biggest whole number possible using the RoundUp()
function.