How to Exit Sub in VBA
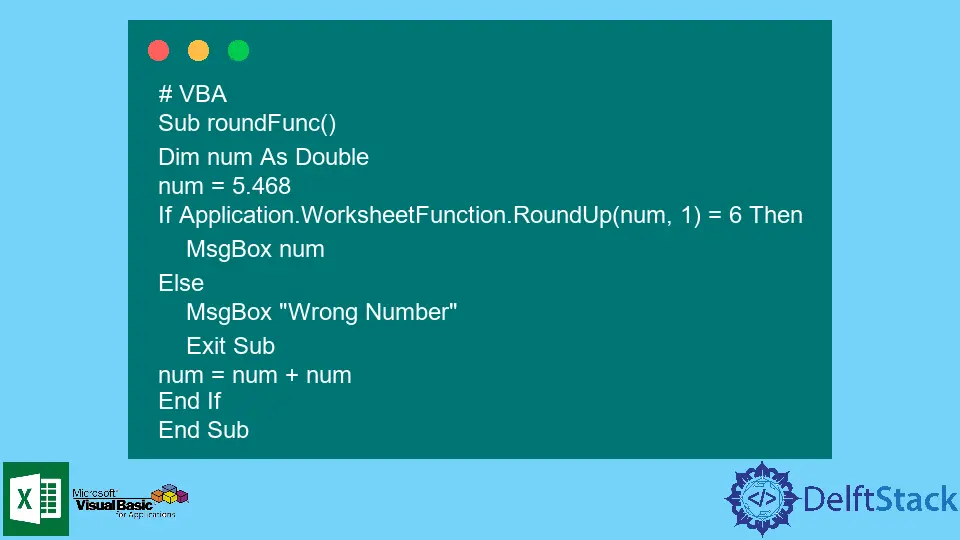
We will introduce different methods to exit sub in VBA with examples.
Use Exit Sub Statement in VBA
While working with Sub in VBA, we may want to exit it or stop it from executing further if there is an error or the user has provided the wrong input. We can also use the exit sub to terminate the sub at any time.
In VBA, each line is executed one by one, so if we have achieved the result or the desired response at a certain point of execution is not as we have wanted, we can use the exit sub to terminate the sub.
There are many situations in which we can use this function. Let’s go through an example and try to exit the sub in the middle of the code.
Example Code:
# VBA
Sub roundFunc()
Dim num As Double
num = 5.468
Exit Sub
MsgBox Application.WorksheetFunction.RoundUp(num, 1)
End Sub
From the above example, I have added the exit sub code before the message box, and when we run this code, no message box appears.
Let’s have another example using the if-else
statement. If the statement is true
, it’ll execute the code until the end. Otherwise, it will break.
Example Code:
# VBA
Sub roundFunc()
Dim num As Double
num = 5.468
If Application.WorksheetFunction.RoundUp(num, 1) = 6 Then
MsgBox num
Else
MsgBox "Wrong Number"
Exit Sub
num = num + num
End If
End Sub
Output:
When we are working with important data and want to make sure that if there is any error or any of the input is wrong, we need to exit the sub immediately to ensure there is no data loss or there is no wrong data inside the company data.