How to Create Variable Range in VBA
- Understanding Variable Ranges in VBA
- Method 1: Using Named Ranges
- Method 2: Dynamic Ranges with Last Row
- Method 3: User Input for Range Selection
- Conclusion
- FAQ
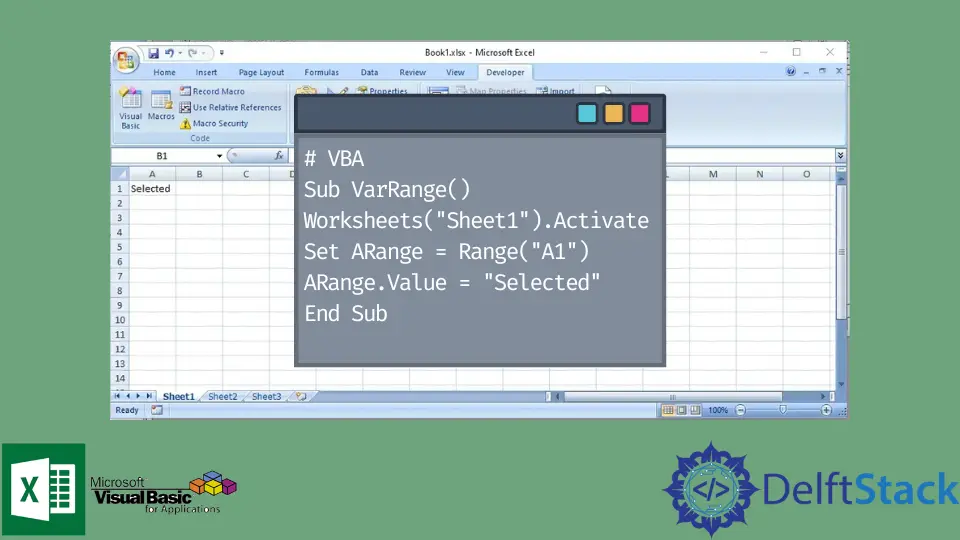
Creating a variable range in VBA (Visual Basic for Applications) can be a powerful tool for automating tasks in Excel. By using variable ranges, you can dynamically adjust your data selection based on user input or data changes within your spreadsheet. This flexibility allows for more efficient data manipulation and reporting.
In this article, we will demonstrate how to create variable ranges in VBA, providing you with practical examples and clear explanations. Whether you’re a beginner or an experienced Excel user, understanding how to set up variable ranges will enhance your VBA skills and streamline your workflow.
Understanding Variable Ranges in VBA
Variable ranges in VBA allow you to define a range of cells dynamically, rather than hardcoding specific cell references. This is particularly useful when working with data that may change in size or when you want to apply the same operations to different sets of data without rewriting your code. The main advantage of using variable ranges is that they make your code more adaptable and easier to maintain.
To create a variable range, you typically use the Range
object in VBA, combined with variables that hold the cell references or row and column numbers. This gives you the ability to manipulate data based on the current state of your worksheet.
Method 1: Using Named Ranges
One effective way to create variable ranges in VBA is by using named ranges. Named ranges allow you to define a specific area in your worksheet that can be referenced easily throughout your code. Here’s how to do it:
Sub CreateNamedRange()
Dim myRange As Range
Set myRange = ThisWorkbook.Sheets("Sheet1").Range("A1:A10")
ThisWorkbook.Names.Add Name:="MyData", RefersTo:=myRange
End Sub
Output:
Named range 'MyData' created for cells A1 to A10
In this example, we define a range from A1 to A10 on “Sheet1” and assign it a name, “MyData.” This named range can now be used throughout your VBA project, making it easier to reference this specific set of cells without needing to remember the exact address. If the data size changes, you can simply adjust the named range in the Excel interface, and your code will still work seamlessly. Named ranges also improve the readability of your code, making it clear what data you are working with.
Method 2: Dynamic Ranges with Last Row
Another common method for creating variable ranges in VBA is to determine the last row of data in a column and then use that to define your range. This is particularly useful for datasets that may grow or shrink over time. Here’s how you can implement this:
Sub DynamicRange()
Dim lastRow As Long
Dim myRange As Range
lastRow = ThisWorkbook.Sheets("Sheet1").Cells(Rows.Count, 1).End(xlUp).Row
Set myRange = ThisWorkbook.Sheets("Sheet1").Range("A1:A" & lastRow)
End Sub
Output:
Dynamic range created from A1 to the last row of data in column A
In this code, we first find the last row of data in column A using the End(xlUp)
method. This technique allows us to dynamically set the range from A1 to the last row, regardless of how many rows of data are present. This is particularly useful for reports or data analysis tasks where the number of entries can vary. By using this method, you ensure that your code is robust and can handle changes in data size without any additional modifications.
Method 3: User Input for Range Selection
Sometimes, you might want to allow users to select the range they want to work with. This can be done using the Application.InputBox
method, which prompts the user for input. Here’s how to implement this:
Sub UserInputRange()
Dim myRange As Range
On Error Resume Next
Set myRange = Application.InputBox("Select a range:", Type:=8)
On Error GoTo 0
If Not myRange Is Nothing Then
MsgBox "You selected the range: " & myRange.Address
Else
MsgBox "No range selected."
End If
End Sub
In this example, the Application.InputBox
method is used to prompt the user to select a range. The Type:=8
argument specifies that the input should be a range. If the user selects a range, a message box displays the address of the selected range. If no range is selected, a different message is shown. This method is great for creating interactive applications where users can specify the data they want to work with, making your VBA scripts more user-friendly.
Conclusion
In this article, we explored various methods to create variable ranges in VBA, including named ranges, dynamic ranges based on the last row, and user input. Each method offers unique advantages, allowing you to choose the best approach based on your specific needs. By mastering these techniques, you can enhance your Excel automation projects and improve your overall productivity. Variable ranges not only make your code more flexible but also help you manage data more effectively. Start experimenting with these methods today and see how they can transform your VBA experience.
FAQ
- What is a variable range in VBA?
A variable range in VBA is a dynamically defined set of cells that can change based on user input or data updates.
-
How can I create a named range in VBA?
You can create a named range in VBA using theNames.Add
method, assigning a specific range of cells a name for easier reference. -
What is the purpose of finding the last row in a column?
Finding the last row allows you to create dynamic ranges that adjust based on the actual amount of data present in your worksheet. -
Can I allow users to select ranges in my VBA code?
Yes, you can use theApplication.InputBox
method to prompt users to select a range, making your code more interactive. -
How does using variable ranges improve my VBA code?
Variable ranges make your code more adaptable, easier to read, and maintain, allowing it to handle changes in data size without additional modifications.