How to Count Rows in VBA
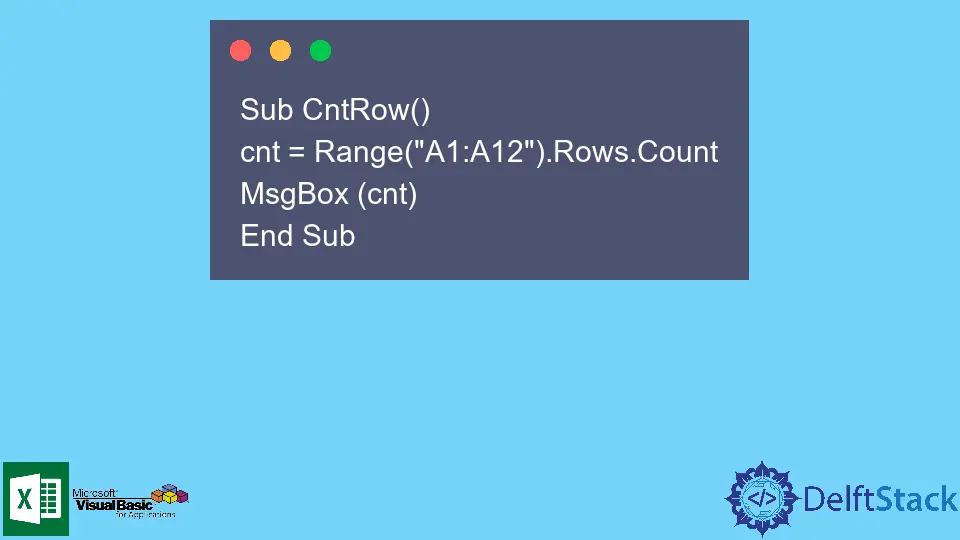
Counting rows in VBA is a fundamental skill for anyone working with Excel automation. Whether you’re a beginner or an experienced developer, understanding how to efficiently count rows can significantly enhance your productivity.
This article will guide you through various methods to count rows in VBA, helping you manage your data more effectively. From simple techniques to advanced methods, we will cover everything you need to know. So, let’s dive into the world of VBA and learn how to count rows like a pro!
Using the Count Property
One of the simplest ways to count rows in VBA is by using the Count
property of a range. This method is straightforward and works well when you want to count all the rows in a specified range. Here’s how it works:
Sub CountRowsUsingCountProperty()
Dim rowCount As Long
rowCount = Worksheets("Sheet1").Range("A1:A100").Rows.Count
MsgBox "Total Rows: " & rowCount
End Sub
In this code, we define a subroutine named CountRowsUsingCountProperty
. We declare a variable rowCount
to store the total number of rows. The Range("A1:A100").Rows.Count
counts the number of rows in the specified range. Finally, we display the total row count in a message box. This method is efficient for counting rows within a defined range and is particularly useful when working with specific data sets.
Output:
Total Rows: 100
This method is beneficial when you know the exact range of data you want to analyze. However, if your data might change or expand, you may want to use a dynamic approach to count rows.
Using the End Property
Another effective method for counting rows in VBA is to use the End
property. This technique is particularly useful when you want to count all the used rows in a column without specifying a fixed range. Here’s how you can do it:
Sub CountRowsUsingEndProperty()
Dim lastRow As Long
lastRow = Worksheets("Sheet1").Cells(Rows.Count, 1).End(xlUp).Row
MsgBox "Total Used Rows: " & lastRow
End Sub
In this example, we create a subroutine called CountRowsUsingEndProperty
. We declare a variable lastRow
to hold the number of used rows. The Cells(Rows.Count, 1).End(xlUp).Row
line finds the last used row in column A by starting from the bottom of the worksheet and moving up. This method is particularly useful for dynamic datasets where the number of rows may vary.
Output:
Total Used Rows: 50
Using the End
property is a flexible way to count rows because it automatically adjusts to the current data in your worksheet. This method is ideal for situations where your data set changes frequently.
Counting Rows with Criteria
Sometimes, you may need to count rows based on specific criteria. For this, you can use the CountIf
function in VBA. This method allows you to count rows that meet certain conditions, making it a powerful tool for data analysis. Here’s an example:
Sub CountRowsWithCriteria()
Dim criteriaCount As Long
criteriaCount = Application.WorksheetFunction.CountIf(Worksheets("Sheet1").Range("A1:A100"), "Passed")
MsgBox "Total Rows with Criteria: " & criteriaCount
End Sub
In this code snippet, we define a subroutine named CountRowsWithCriteria
. We declare a variable criteriaCount
to store the count of rows that meet the specified criteria. The CountIf
function checks the range “A1:A100” for the value “Passed”. The result is displayed in a message box.
Output:
Total Rows with Criteria: 30
This method is particularly useful for filtering data based on specific conditions, allowing you to quickly analyze your dataset. Whether you’re counting sales figures, grades, or any other data type, this approach can save you time and effort.
Conclusion
Counting rows in VBA is an essential skill that can significantly enhance your data management capabilities in Excel. Whether you choose to use the Count
property, the End
property, or count rows based on specific criteria, each method has its unique advantages. By mastering these techniques, you can streamline your workflow and make your data analysis more efficient. Remember, the key to effective data management is knowing which method to use for your specific needs. Now that you have a solid understanding of how to count rows in VBA, you’re well on your way to becoming a more proficient Excel user.
FAQ
-
How do I count all rows in a worksheet using VBA?
You can use theCount
property or theEnd
property to count all rows in a worksheet. -
Can I count rows based on multiple criteria?
Yes, you can use theCountIfs
function to count rows based on multiple criteria. -
What if my data range changes frequently?
Using theEnd
property is ideal for counting rows in a dynamic dataset that changes frequently.
-
Is it possible to count rows in a specific column?
Yes, you can specify a column in your range when using methods likeCount
orCountIf
. -
Can I display the row count in a cell instead of a message box?
Yes, you can assign the row count to a cell instead of displaying it in a message box by usingWorksheets("Sheet1").Range("B1").Value = rowCount
.