How to Clear Clipboard in VBA
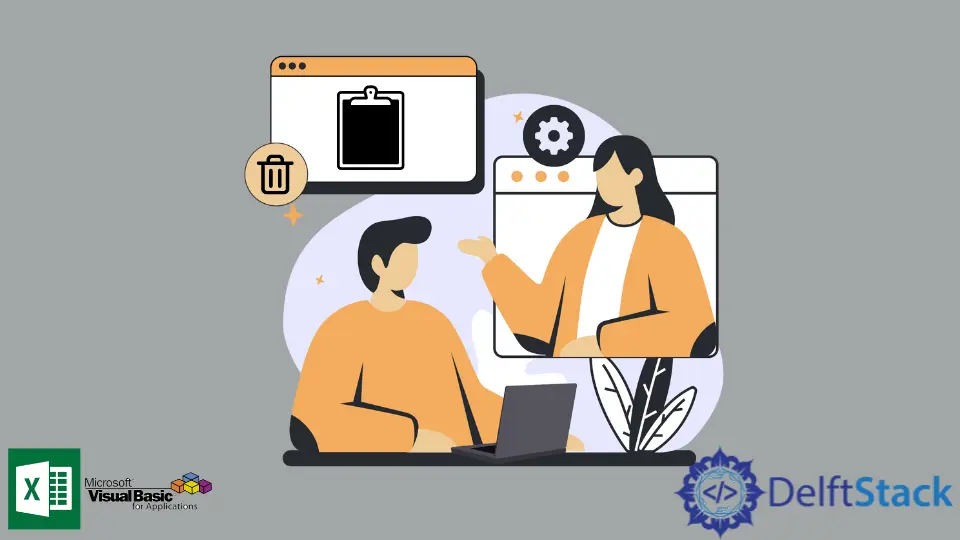
This article will discuss how we can clear a clipboard in VBA.
Excel VBA Command to Clear the Clipboard
In Excel, we can deal with many data that we conveniently move around through copy and paste. But doing this will clutter the clipboard, or if we are using a public platform while working, there is a chance of misuse of your data.
We can avoid this issue by clearing the clipboard after every paste, but this is a tedious task. Normally in VBA, we can escape this by avoiding copying and pasting and using other mediums to transfer the data, but we can’t deny that copying and pasting is the most convenient option.
Erasing data from the clipboard is not a difficult task in VBA. Use the trouble-free one line of code below.
Code:
# vba
Sub ClearClipboard()
Application.CutCopyMode = False
End Sub
Output:
We can place this after we are done pasting, which will erase all cache from our clipboard. The code can be added just about the end of our macro.
The other option that we can avail ourselves of can enter the code after each paste in our code. This will clear the clipboard before the next copy and paste.
We can also clear the windows clipboard in excel by using VBA. This can be done by using the Empty Clipboard Function.
Code:
# vba
Sub ClearClipboard()
Range("A1"A4").Copy Destination:=Worksheets("Sheet2").Range("A1")
Application.CutCopyMode = False
End Sub
When we have entered the code, progress to Sub ClearClipboard()
.
Output: