How to Call Userform Initialize in VBA
- Understanding UserForm_Initialize
- Example of UserForm_Initialize in VBA
- Customizing UserForm Appearance
- Adding Dynamic Data to UserForms
- Conclusion
- FAQ
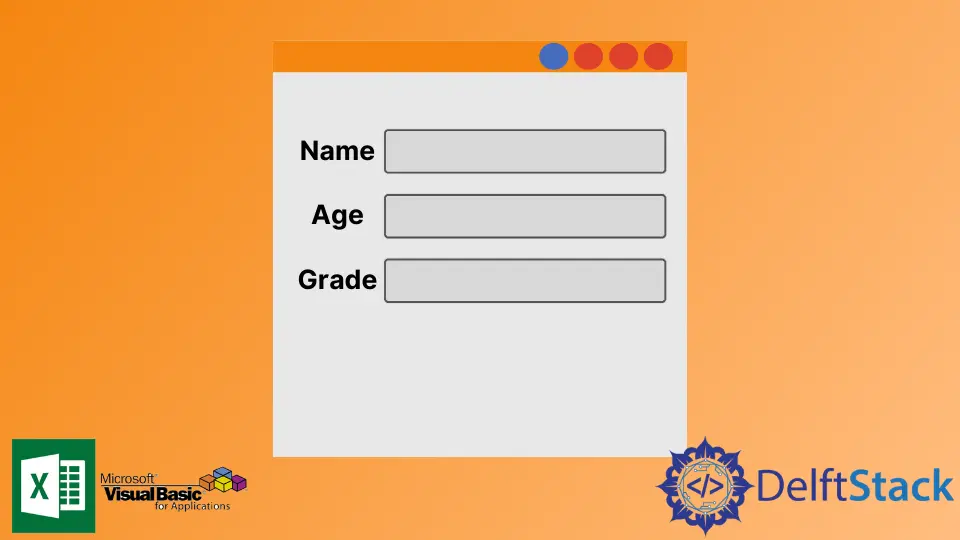
Visual Basic for Applications (VBA) is a powerful tool for automating tasks in Microsoft Office applications. One of the essential features of VBA is the UserForm, which allows users to create custom forms for data entry and interaction. Understanding how to call the UserForm_Initialize
event is crucial for ensuring that your forms load with the necessary data and settings.
In this article, we will explore how to effectively use the UserForm_Initialize
event in VBA. We will cover the key concepts, provide practical examples, and explain how this event can enhance your user experience in Excel, Access, and other Office applications. Whether you are a beginner or an experienced developer, this guide will help you master this important aspect of VBA.
Understanding UserForm_Initialize
The UserForm_Initialize
event is triggered when a UserForm is loaded. This is the perfect opportunity to set initial values, populate controls, and prepare the form for user interaction. By using this event, developers can ensure that the UserForm is user-friendly and tailored to the specific needs of their application.
To effectively utilize this event, you need to understand how to access it within your VBA code. Here’s a simple example of how to implement the UserForm_Initialize
event in a UserForm.
Example of UserForm_Initialize in VBA
Private Sub UserForm_Initialize()
Me.TextBox1.Value = "Enter your name"
Me.ComboBox1.AddItem "Option 1"
Me.ComboBox1.AddItem "Option 2"
Me.ComboBox1.AddItem "Option 3"
End Sub
In this example, when the UserForm is initialized, the first text box is pre-filled with a prompt, and a ComboBox is populated with three options. This provides a clear starting point for users and enhances their interaction with the form.
The Me
keyword refers to the current instance of the UserForm. By using Me.TextBox1.Value
, we set the default text of the TextBox. Similarly, the ComboBox1.AddItem
method allows us to add items to the ComboBox dynamically. This creates a more interactive and engaging UserForm experience.
Customizing UserForm Appearance
Another powerful aspect of the UserForm_Initialize
event is the ability to customize the appearance of your UserForm. This can include changing colors, fonts, and visibility of controls based on specific criteria. Here’s how you can achieve this:
Private Sub UserForm_Initialize()
Me.Caption = "User Information"
Me.BackColor = RGB(240, 240, 240)
Me.Label1.Caption = "Please fill in your details"
Me.TextBox1.Visible = True
End Sub
In this code, we set the title of the UserForm to “User Information” and change its background color to a light gray. The label caption is updated to guide users, and we ensure that the TextBox is visible. Such customization not only enhances the visual appeal but also improves usability.
The RGB
function allows you to define colors using their red, green, and blue components, making it easy to create a visually appealing UserForm. By adjusting these properties, you can create a UserForm that aligns with your application’s branding and user experience goals.
Adding Dynamic Data to UserForms
One of the most powerful features of the UserForm_Initialize
event is its ability to populate controls with dynamic data. This is particularly useful when you want to display information from a database or a worksheet. Here’s an example of how to load data from an Excel worksheet into a UserForm:
Private Sub UserForm_Initialize()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Data")
Dim i As Integer
For i = 1 To ws.Cells(Rows.Count, 1).End(xlUp).Row
Me.ListBox1.AddItem ws.Cells(i, 1).Value
Next i
End Sub
In this example, we open a worksheet named “Data” and loop through its first column to populate a ListBox with values. This allows users to select from a list of data that is automatically updated based on the contents of the worksheet.
Using the Cells
method helps you access specific cells in the worksheet, and the End(xlUp)
method finds the last filled cell in the column. This makes your UserForm dynamic and responsive to changes in the underlying data, providing a seamless user experience.
Conclusion
Mastering the UserForm_Initialize
event in VBA can significantly enhance your applications by providing users with a more interactive and tailored experience. By following the examples and techniques discussed in this article, you can effectively initialize your UserForms, customize their appearance, and load dynamic data. Whether you are creating simple forms or complex applications, leveraging the UserForm_Initialize
event will help you build more efficient and user-friendly solutions.
FAQ
-
What is the purpose of the UserForm_Initialize event in VBA?
The UserForm_Initialize event is used to set up initial values and properties for a UserForm when it is loaded. -
How can I populate a ComboBox in a UserForm?
You can use theAddItem
method within the UserForm_Initialize event to add items to a ComboBox. -
Can I change the appearance of a UserForm during initialization?
Yes, you can customize properties like the background color and captions in the UserForm_Initialize event. -
How do I load data from an Excel worksheet into a UserForm?
You can loop through the cells of a worksheet and use theAddItem
method to populate controls like ListBoxes and ComboBoxes. -
Is it possible to make controls visible or invisible in the UserForm_Initialize event?
Yes, you can set theVisible
property of controls to True or False to show or hide them during initialization.