How to Use Any Data Type in TypeScript
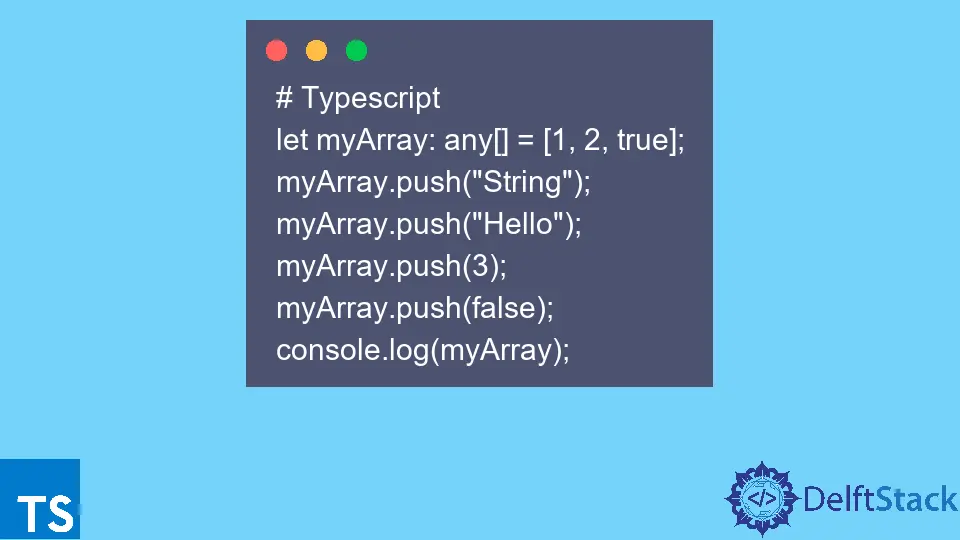
We will introduce how to use the any
data type in Typescript with examples.
Use any
Data Type in TypeScript
When we are working on developing commercial applications, there are situations in which we cannot always know what a user is going to type in a certain field. For this purpose, we need to define variables to be user-friendly and always pass when compiling or the type of the variable being checked.
TypeScript always checks the type of variables and adds some checks during compile time. Most of the time, we can easily define the type of variables, but for some unusual times where a user may enter a number or a string, It can be difficult to manage multiple types for a single variable.
TypeScript provides a new data type that can be used when we are not sure about the type of a variable or what type of data is going to be inserted into the following variable. any
is a data type in TypeScript that we use to define a variable to bypass the type checking and compile-time checking of a variable.
Let’s go through an example and use this data type to declare a variable and then try to pass multiple types of values in the same variable.
Example Code:
# Typescript
let vari: any = 7;
console.log(vari);
vari = "String Passed";
console.log(vari);
vari = false;
console.log(vari)
Output:
As we can see from the above example, we can pass any type of value to a variable that is declared using any
data type. Similar to the variables, we can also define arrays using any
data type.
Let’s have another example where we will create an array using the any
data type.
Example Code:
# Typescript
let myArray: any[] = [1, 2, true];
myArray.push("String");
myArray.push("Hello");
myArray.push(3);
myArray.push(false);
console.log(myArray);
Output:
In the above example, we can insert any type of value inside the array after creating with any data type in TypeScript.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn