How to Enumerate String in TypeScript
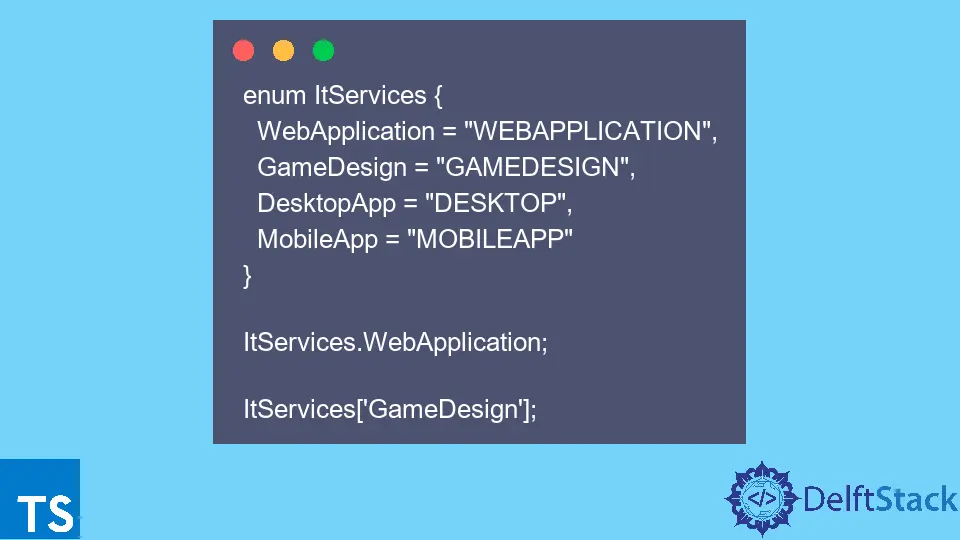
This article will discuss how string enum
works in TypeScript with some examples. We would also learn about the advantages and disadvantages of string enum
in TypeScript.
String Enum
in TypeScript
This article will explore and discuss string enums
in TypeScript. If you are new and unfamiliar with the JavaScript/TypeScript landscape, you might ponder what enums
are.
The enums
keyword offers us to define a finite set of values.
String enums
are just like numeric enums
. The enum
values begin with string values rather than numeric values.
The advantage of using string enums
is that string enums
have better readability. If we debug a program, it is easier to read string values than numeric values.
There is an example of a numeric enum
, but it is represented as a string enum
.
Example: String Enum
Copy
enum ItServices {
WebApplication = "WEBAPPLICATION",
GameDesign = "GAMEDESIGN",
DesktopApp = "DESKTOP",
MobileApp = "MOBILEAPP"
}
ItServices.WebApplication;
ItServices['GameDesign'];
The values as the numeric enum
above have described a string enum
and ItServices
, with the identical values, with the difference that these enum
values are initialized with string literals.
The difference between numeric and string enums
is that numeric enum
values are advanced, while string enum
values need to be individually initialized.
Advantages of Using String Enum
in TypeScript
The advantages of TypeScript for client-side developers are versatile. It is easier to pick up than other alternatives, as you can jump in with a JavaScript background.
TypeScript enums
make the document easier to intend or create a distinct set of cases.
Disadvantages of Using String Enum
in TypeScript
TypeScript’s enums
are very convenient because there’s nothing like an enum
yet in JavaScript, probably because a JavaScript object is very close to an enum
. But if we are writing TypeScript code, the enum
informs us about the read-only state.
However, the TypeScript enum
must be transpired to an object at build time. The output will be a very verbose transpiled output for something that could be simply an object structure.
The developer must choose between a lighter output using an object or a better typing comfort using the enum
type.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn