How to Check if a String Has a Certain Text in TypeScript
-
Check if a String Has a Certain Text Using the
indexOf()
Method in TypeScript -
Check if a String Has a Certain Text Using the
search()
Method in TypeScript -
Check if a String Has a Certain Text Using the
includes()
Method in TypeScript
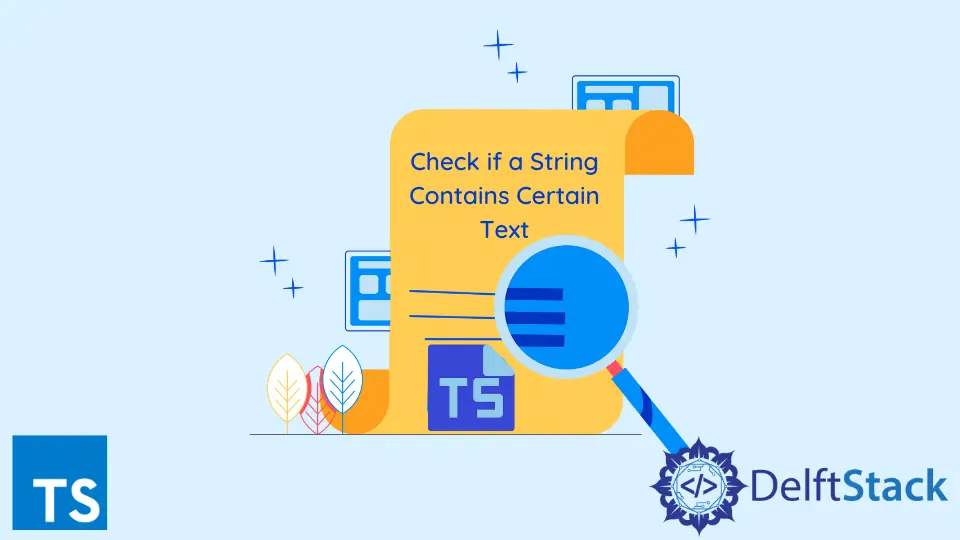
We will introduce how to check if a string contains a substring in TypeScript with examples.
Strings are widely used in every application. While working on some applications, we may want to check if a particular string contains a certain substring in it or not.
This can be used in email detector applications or applications used to filter chats or emails for different purposes.
TypeScript provides three methods that can be used to check whether a string contains a particular substring or text. We will discuss these methods in detail.
Check if a String Has a Certain Text Using the indexOf()
Method in TypeScript
The indexOf()
method is used to check whether a string contains another string or not. An example code using this method is shown below.
# typescript
let str1 = "Hello";
let str2 = "Hello! Good Morning";
if(str1.indexOf(str2)){
console.log("String 2 contains string 1!")
}
Output:
As we can see from the above example, str2
contains the word Hello
. And the result is also the same, as we can see by comparing both strings.
Check if a String Has a Certain Text Using the search()
Method in TypeScript
TypeScript provides another method, search()
, that can be used to search if a string contains the substring. Let’s use this method in an example in which we will define two variables and compare them, as shown below.
# typescript
let str1 = "Hello";
let str2 = "Hello! Good Morning";
if(str1.search(str2)){
console.log("String 2 contains string 1!")
}
Output:
As we can see from the above example, we got the same result as the previous example using the indexOf()
method.
Check if a String Has a Certain Text Using the includes()
Method in TypeScript
TypeScript provides another method, includes()
, to search if a string contains the substring. Let’s use this method in an example in which we will define only one variable and try to search for a specific string from it, as shown below.
# typescript
let str2 = "Hello! Good Morning";
if(str2.includes('Hello')){
console.log("String 2 contains string 1!")
}
Output:
As we can see from the above example, we got the same result as the above two methods.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn