How to Sleep in TypeScript
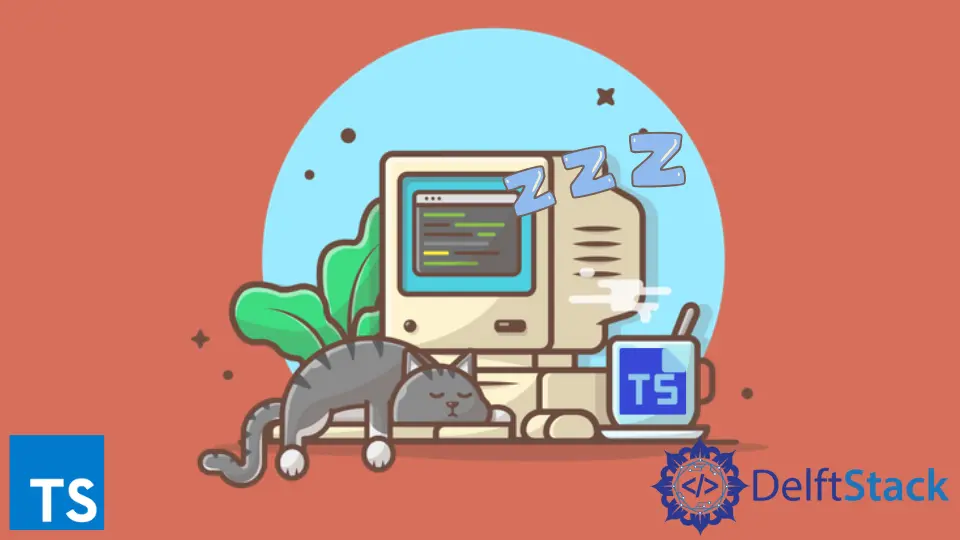
While implementing business logic, it is often needed to add a delay to a function or sleep for some time waiting for some API call.
This tutorial will discuss different ways and concepts behind sleeping a thread in TypeScript.
Use Blocking to Sleep a Thread in TypeScript
Blocking can be used to wait for some time in a synchronous manner. The following code sample demonstrates how a thread can be blocked, so execution happens after some seconds.
function delayBlocking(milliseconds: number){
const timeInitial : Date = new Date();
var timeNow : Date = new Date();
for ( ; timeNow - timeInitial < milliseconds; ){
timeNow = new Date();
}
console.log('Sleep done!');
}
console.log('Starting, will sleep for 5 secs now');
delayBlocking(5000);
console.log('Normal code execution continues now');
Output:
Starting, will sleep for 5 secs now
Sleep done!
Normal code execution continues now
The above code segment will sure stop execution for some time, but sometimes this is not the desired effect as for a large delay, the entire code will be blocked.
Use Promises to Sleep in TypeScript
The setTimeout
function is used to resolve a Promise
after some delay. This method can be chained with then
or async...await
. The following code segment shows behavior with then
.
function delay(milliseconds : number) {
return new Promise(resolve => setTimeout( resolve, milliseconds));
}
console.log('Starting, will sleep for 5 secs now');
delay(5000).then(() => console.log('Normal code execution continues now') );
With async..await
it can be implemented according to the following code segment-
function delay(milliseconds : number) {
return new Promise(resolve => setTimeout( resolve, milliseconds));
}
( async() => {
console.log('Starting, will sleep for 5 secs now');
await delay(5000);
console.log('Normal code execution continues now');
})();