How to Record in TypeScript
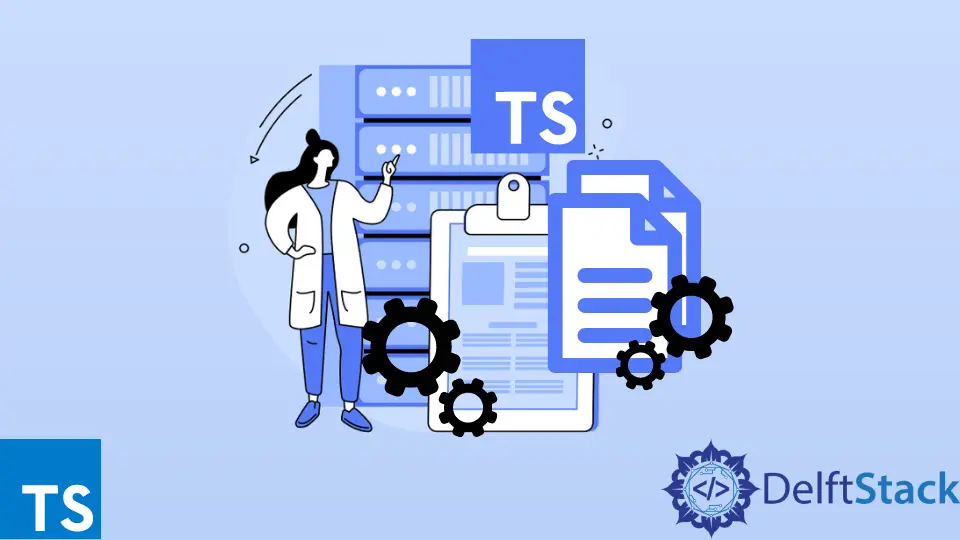
This article will introduce records
in TypeScript and how it works.
Record
in TypeScript
Record
in TypeScript is the best way to ensure stability while testing or implementing more complex data types. They impose strong values and allow you to create custom interfaces for the values.
This idea is confusing, but let’s see how it works in practice. A Record
is a utility type - in which TypeScript is primarily defined to help with a specific problem.
const students = {
"11-12-13" : { firstName: "Petr", lastName: "David" },
"14-15-16" : { firstName: "James", lastName: "Andrew" },
"16-17-18" : { firstName: "Julia", lastName: "Saggy" }
}
In this following code, we have data set that has an ID for its key, type string.
All the values have the same format. That is, we have firstName
and lastName
.
For this code, a Record
is the best utility type. We can define our data structure type as follows.
type students = {
firstName: string,
lastName: string
}
const class:Record<string, students> = {
"21-22-23" : { firstName: "David", lastName: "Miller" },
"24-25-26" : { firstName: "John", lastName: "Smith" },
"27-28-29" : { firstName: "Joe", lastName: "Peterson" }
}
This Record
takes the form Record<K, T>
, where K
is the type of the key, and T
is the type of the values.
Previously, we elaborated a new type of students
for our values and set our keys to type string.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn