How to Write console.log Wrapper for Angular 2 in TypeScript
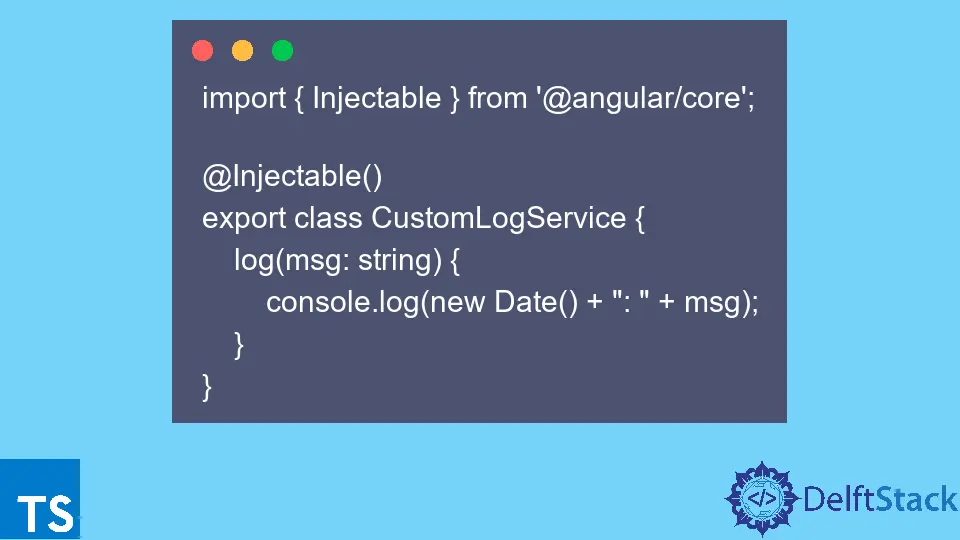
Logging is very important for applications, which helps debug purposes and know any application issues in production. Angular is all about services and can be injected into other components so that a logging service can be created, which acts as a wrapper for the console.log
function.
The logging service can be extended to further actions with logs, like sending them to a remote server for analytics or a database. This tutorial will demonstrate how services in Angular can be used to make a wrapper for the console.log
function in TypeScript.
Write console.log
Wrapper for Angular 2 in TypeScript
As Angular consists of services, one can create a new logging service. This service can be later extended for further use cases like analytics and observability.
services/log.service.ts
import { Injectable } from '@angular/core';
@Injectable()
export class CustomLogService {
log(msg: string) {
console.log(new Date() + ": " + msg);
}
}
Thus CustomLogService
acts as a wrapper around console.log
and can be used in other Angular components. For injecting the CustomLogService
class, we can define a component that an HTML file can use.
tests/log.component.html
<button (click)="wrapLog()">Log</button>
tests/log.component.ts
import { Component } from "@angular/core";
import { CustomLogService } from "../services/log.service";
@Component({
selector: "log-comp",
templateUrl: "./log.component.html"
})
export class LogComponent {
constructor(private logger: CustomLogService) {}
wrapLog(): void {
this.logger.log("A custom log method");
}
}
A further app.module.ts
needs to be defined.
app.module.ts
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { AppComponent } from "./app.component";
import { CustomLogService } from "./services/log.service";
import { LogComponent } from "./tests/log.component";
@NgModule({
imports: [BrowserModule],
declarations: [AppComponent, LogComponent],
bootstrap: [AppComponent, LogComponent],
providers: [CustomLogService]
})
export class AppModule {}
Now the selector log-comp
defined in tests/log.component.ts
can be used as <log-comp></log-comp>
to display an HTML button, which when clicked, logs to the console - A custom log method
as defined in tests/log.component.ts
.
Output:
Here’s the demo for the complete source code.