How to Have Multiline Strings in TypeScript
- Use Template Strings to Form Multi-line Strings in TypeScript
-
Use
Array.join()
to Form Multiline Strings in TypeScript
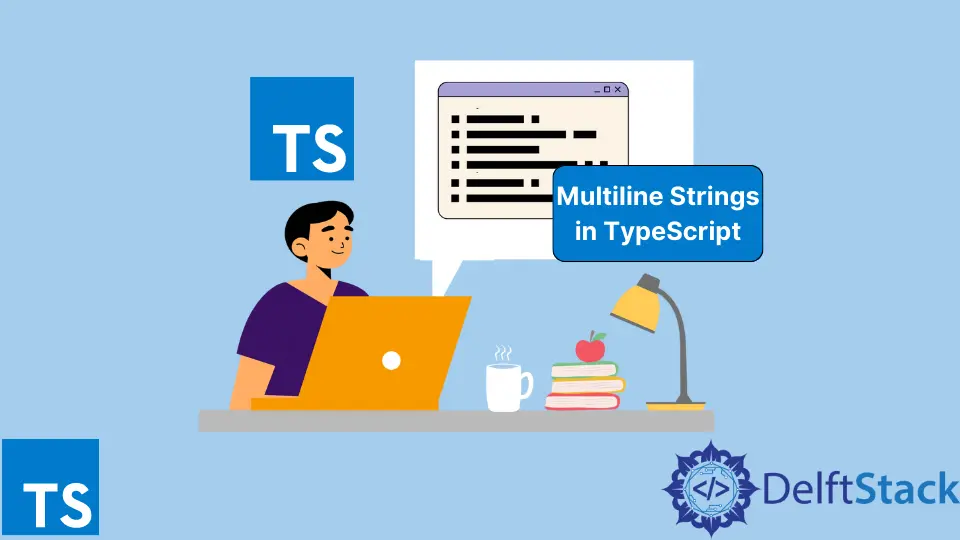
Strings are important for every programming language, and TypeScript is no different in this regard. Apart from single line strings, TypeScript supports multi-line strings where a new line separates strings.
This article will demonstrate different methods by which multi-line strings can be generated in TypeScript.
Use Template Strings to Form Multi-line Strings in TypeScript
Template strings start with a backtick and can be further used for string interpolations and can be used to evaluate expressions at runtime while forming the string. However, template strings can also be used to generate multi-line strings, or it maintains the same formatting as the string.
The following code block will show how template strings can be used to generate multi-line strings in TypeScript.
var multiLineString : string = `This is
a nice day to
write some
multi-line
strings.`
console.log(multiLineString);
Output:
"This is
a nice day to
write some
multi-line
strings."
Thus the output log comes exactly as formatted as the input. This is unlike the regular "
quotes used while writing strings which won’t preserve the formatting as on input.
var multiLineString : string = "This \
won't be \
formatted as a \
multi-line \
string."
console.log(multiLineString);
Output:
"This won't be formatted as a multi-line string."
Use Array.join()
to Form Multiline Strings in TypeScript
The Array.join()
method is another way to form a multi-line string from an array of strings in TypeScript. The following code block shows how this can be achieved.
var stringArr : Array<string> = [ 'This', 'is', 'a' , 'multi-line', 'string'] ;
var multiString : string = stringArr.join('\n');
console.log(multiString);
Output:
"This
is
a
multi-line
string"