How to Initialize an Array in TypeScript
- Initialize an Array of Certain Types in TypeScript
- Use Named Parameters to Initialize an Array in TypeScript
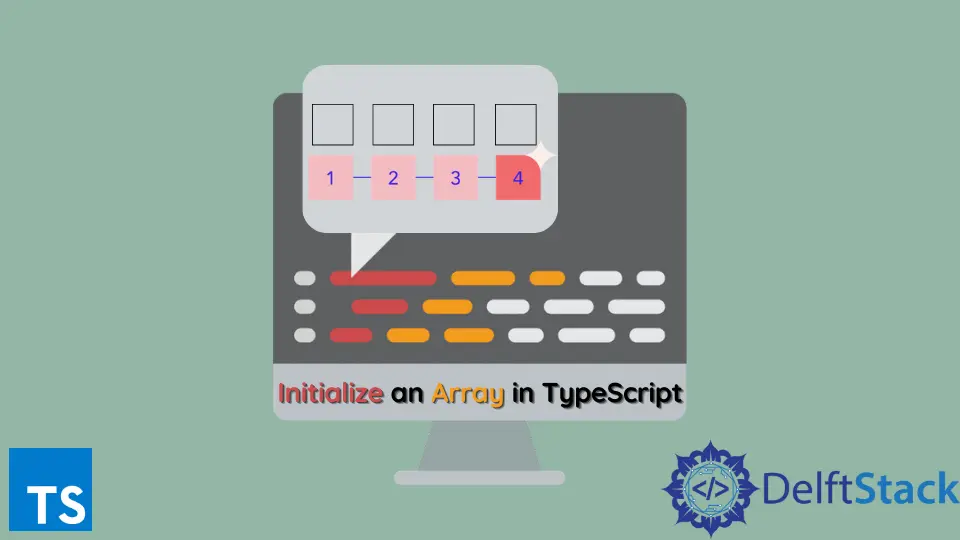
Arrays in TypeScript or JavaScript are data structures for storing continuous memory segments. Arrays are extremely useful and often used in almost all applications.
This tutorial will demonstrate how an array can be initialized in TypeScript.
Initialize an Array of Certain Types in TypeScript
An array in TypeScript can be initialized using the empty array value and Array
type, assigned to a custom type.
class Product {
name : string;
quantity : number;
constructor(opt : { name? : string, quantity? : number}){
this.name = opt.name ?? 'default product';
this.quantity = opt.quantity ?? 0;
}
}
var products : Array<Product> = [];
products.push( new Product({name : 'pencil', quantity : 10}) )
products.push( new Product({name : 'pen', quantity : 4}) )
console.log(products);
Output:
[Product: {
"name": "pencil",
"quantity": 10
}, Product: {
"name": "pen",
"quantity": 4
}]
Instead of the var products : Array<Product> = [];
, one can also use the var products : Product[] = [];
, and obtain the same functionality and the typing support by TypeScript.
The arrays can also be of the type interface
.
interface Product {
name: string;
quantity : number;
}
var products : Array<Product> = [];
var prod : Product[] = []
products.push({name : 'pencil', quantity : 10});
products.push({name : 'pen', quantity : 4});
console.log(products);
Output:
[{
"name": "pencil",
"quantity": 10
}, {
"name": "pen",
"quantity": 4
}]
Use Named Parameters to Initialize an Array in TypeScript
An array can also be initialized inside a class using the Array()
class. Then the class variable can be directly used to set elements in the array or push elements.
class Car {
model : string;
buildYear : number;
constructor( opt : { model? : string, buildYear? : number }){
this.model = opt.model ?? 'default';
this.buildYear = opt.buildYear ?? 0;
}
}
class Factory {
cars : Car[] = new Array();
}
var factory = new Factory();
factory.cars = [ new Car({model : 'mercedes', buildYear : 2004}), new Car( { model : 'BMW', buildYear : 2006}) ];
console.log(factory.cars);
Output:
[Car: {
"model": "mercedes",
"buildYear": 2004
}, Car: {
"model": "BMW",
"buildYear": 2006
}]