TypeScript in Operator
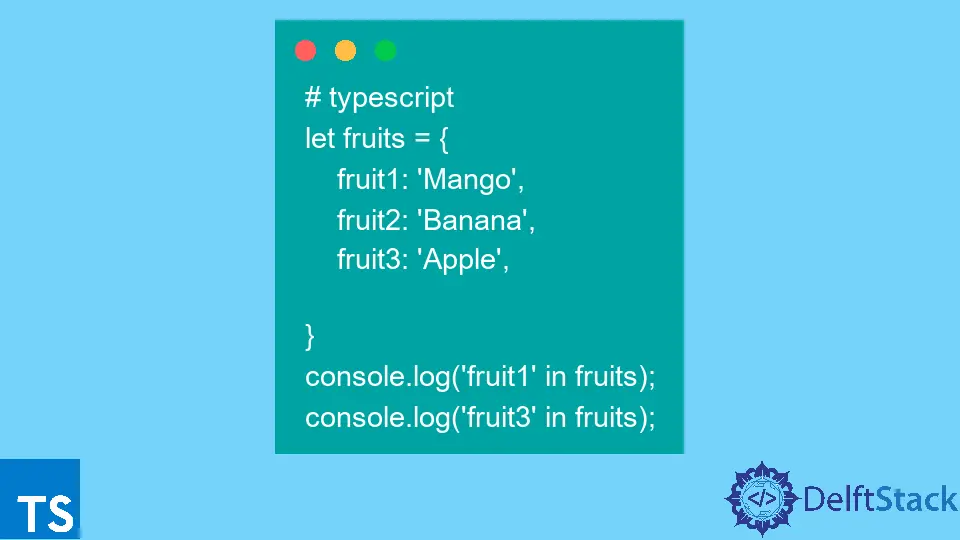
This article will introduce the in
operator in TypeScript and how we can use it with examples.
TypeScript in
Operator
While working with objects and arrays, there are many situations in which we need to check for the object or array properties. For this purpose, JavaScript provides the in
operator that can be used to check whether a property exists in a certain object or not.
The syntax of the in
operator is shown below.
# typescript
x in thisObject;
If x
exists in the object thisObject
, this expression will return true. If x
does not exist in the object thisObject
, this expression will return false.
Now, let’s go through an example in which we will create an object of fruits
and use the in
operator to check whether mango
exists in the array or not, as shown below.
# typescript
let fruits = {
fruit1: 'Mango',
fruit2: 'Banana',
fruit3: 'Apple',
}
console.log('fruit1' in fruits);
console.log('fruit3' in fruits);
Output:
As we can see from the above example, using the in
operator, we can easily check any property from the object. We can also use this operator on an array, but it will only check for the index in an array.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn