TypeScript forEach() Loop
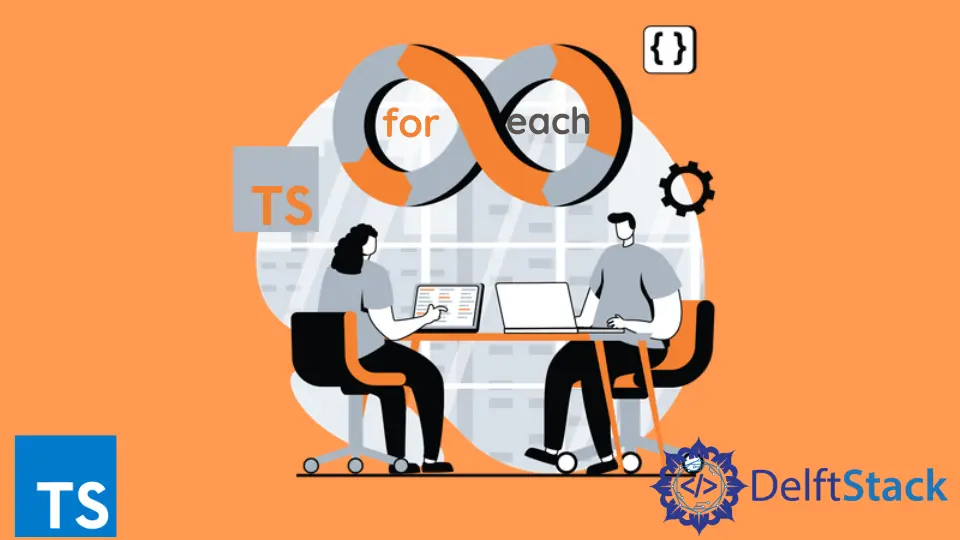
We will introduce how to use the forEach()
loop and discuss its advantages and disadvantages.
the forEach()
Loop in TypeScript
There are many situations in which we may need to iterate through arrays and perform some functions on each element of that array. In TypeScript, we can use some loops for this function.
The forEach()
loop can be used to iterate through an array. We will use this loop to iterate each item in an array.
We can also use it in TypeScript data types, for example, Arrays, Maps, Sets. etc. This method helps arrange elements in an array.
Syntax:
The forEach()
loop is mentioned below.
array.forEach(callback[, thisObject]);
The forEach()
loop is executed to provide the callback
one time for each presented element according to ascending order in an array.
Parameter Details
A callback
is a function used to evaluate every element. This function of the callback
is discussed in the three arguments below.
- The
Element Value
is the value of the currently used item. - The
Element index
is the current element of the index in an array that is processed. - An
Array
is a method in the being of the iterated inforEach()
.
thisObject
is to be used when we have to execute the callback
method.
Return Value
The created array will be returned.
let Brands = ['Iphone', 'Samsung', 'Huawei'];
let Store: any[] = [];
Brands.forEach(function(item){
Store.push(item)
});
console.log(Store);
Output:
Example with number:
var quantity = [5, 10, 15];
quantity.forEach(function (stock) {
console.log(stock);
});
Output:
the Advantages of forEach()
in TypeScript
This is only one advantage mentioned here, as given below.
- It is a more up-to-date way with lesser code to emphasize an array.
- An
Iterator
, index of the item, and array to iterate are its parameters.
the Disadvantages of forEach()
in TypeScript
- It is slower than the conventional circle in execution.
- The
break
statement can’t be utilized due to thecallback
function. - The keyword can’t be used in the
callback
function, and it may go toward the incorrect result.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn