How to Use the declare Keyword in TypeScript
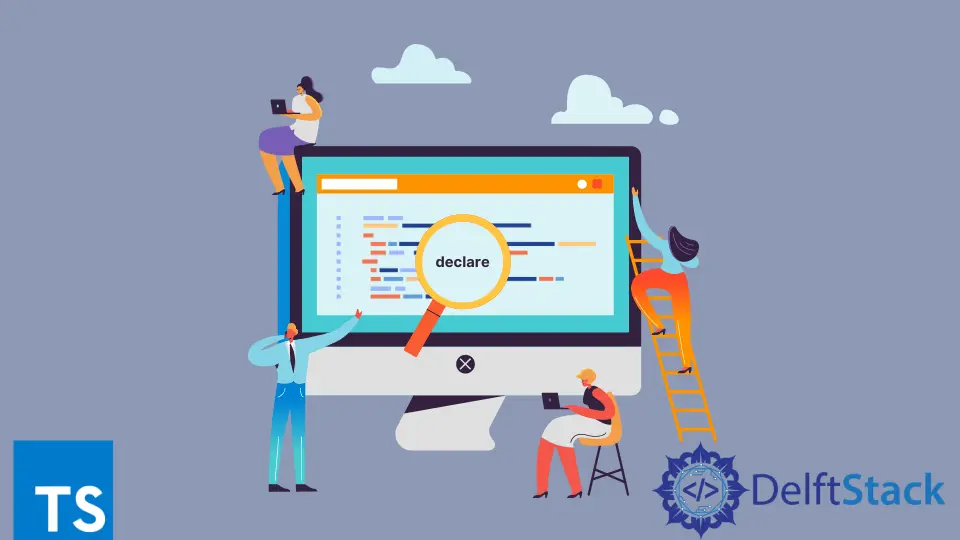
This article explains the purpose of the declare
keyword in TypeScript with real-world coding examples and implementation done among the developer’s community. It will also provide the readers with the advantages of using the declare
keyword in TypeScript.
Let’s dive into this tutorial and start learning.
Use the declare
Keyword in TypeScript
The TypeScript declare
keyword is utilized for the Ambient declaration of methods or variables. Ambient Declarations can also be considered an import keyword, informing the compiler that the source is present in another file.
We mostly use Ambient declarations in TypeScript for using the third-party libraries of Node
, jQuery
, JavaScript
etc. Using the declare
keyword directly for integrating these libraries in our code will decrease the chance of error in our TypeScript code.
The following is the syntax used for the declare
keyword.
declare var variableName;
declare module nameOfModule{// Body of module
};
Let us consider the following example of code in which we have third-party JavaScript code that contains the value of some variables, but we don’t require changing them; instead, we want to use it to use the declare
keyword for this purpose.
For example, we have a file containing some useful variables, but the file is written in JavaScript. Since we want to use it inside the TypeScript without rewriting the code since it is time-consuming, we could use the declare
keyword.
Consider the third-party code is below.
// JavaScript Code
var pi_one = 3.1415926535;
var pi_two = 3.14159265358979323846;
var pi_three = 3.141592653589793238462643383279;
//TypeScript Code
declare var pi_one : any ;
console.log("Value of pi is :",pi_one)
Now we can link both the file with HTML code and use them.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>declare keyword</title>
</head>
<body>
</body>
<!-- Javascript Library -->
<script src="C:\Users\computers\Desktop\typescript\first1.js">
</script>
<script src="C:\Users\computers\Desktop\typescript\secons.js">
</script>
</html>
The output for the above code will be below.
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn