How to Convert an Object Into a JSON String in TypeScript
-
Use the
JSON.stringify()
Method to Convert an Object Into a JSON String in TypeScript -
Use
JSON.stringify()
andJSON.parse()
to Convert an Object Into a JSON String in TypeScript
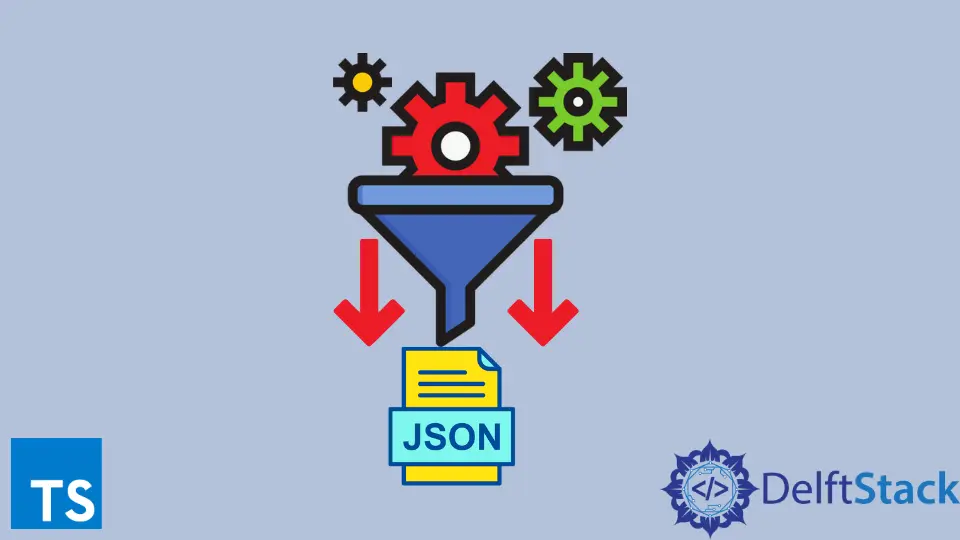
This tutorial will introduce converting a TypeScript object to a JSON string.
Use the JSON.stringify()
Method to Convert an Object Into a JSON String in TypeScript
In TypeScript, we will use the JSON.stringify()
method to turn any object into a JSON string.
Below are some code examples to better understand how these methods work. Let’s consider the person
object, which contains the person’s first and last name.
let person = {
firstName:"Ibrahim",
lastName:"Alvi"
};
console.log(person)
let jsonData = JSON.stringify(person);
console.log(`The person object is : ${person} and it's JSON string is: ${jsonData}`);
Output:
Use JSON.stringify()
and JSON.parse()
to Convert an Object Into a JSON String in TypeScript
Suppose we want to parse it back into the previous object. We use the JSON.parse()
method to parse a JSON string to construct a TypeScript object described by the string.
The code below shows how object data can be saved to the local browser storage by turning it into a JSON string and how it can be retrieved and parsed.
We will use the JSON.stringify()
and JSON.parse()
methods.
// Storing data:
const dataObj = {
name: "Ibrahim",
age: 31,
city: "Karachi"
};
const myJSON = JSON.stringify(dataObj);
localStorage.setItem("testJSON", myJSON);
// Retrieving data:
let text = localStorage.getItem("testJSON");
let obj = JSON.parse(text);
document.getElementById("dataid").innerHTML = obj.name;
Output:
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn