The as Keyword in TypeScript
-
Understanding the
as
Keyword -
Using the
as
Keyword with Interfaces - Type Assertions with Union Types
- Conclusion
- Frequently Asked Questions
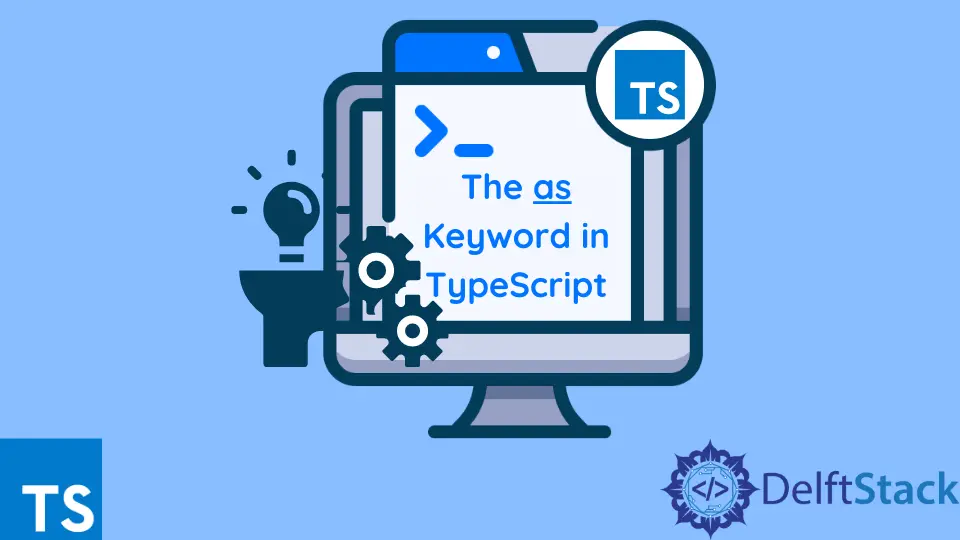
TypeScript, a superset of JavaScript, enhances the development experience by adding static types. One of the essential features of TypeScript is the as
keyword, which plays a significant role in type assertions. By using as
, developers can inform the TypeScript compiler about the type of a variable, allowing for more precise type checking and better code quality.
In this tutorial, we will explore the purpose of the as
keyword in TypeScript, how it can be effectively utilized, and the benefits it brings to your development workflow. Whether you’re a beginner or an experienced developer, understanding the as
keyword will enhance your coding skills and improve your TypeScript projects.
Understanding the as
Keyword
The as
keyword in TypeScript is primarily used for type assertions. Type assertions allow developers to specify a type for a variable, overriding the inferred type. This is particularly useful when you know more about the type of a variable than TypeScript’s type inference system does.
For instance, suppose you have a variable that is inferred as a generic type, but you know it holds a more specific type. By using as
, you can tell TypeScript to treat that variable as the specific type you know it to be. This can help prevent type-related errors and improve code clarity.
Here’s a simple example:
let someValue: any = "Hello, TypeScript!";
let strLength: number = (someValue as string).length;
In this example, someValue
is declared as any
, which means it can hold any type of value. However, when we want to access the length
property, we use as string
to assert that someValue
is indeed a string. This allows TypeScript to understand that we are working with a string, enabling us to safely access its properties.
Output:
12
The output shows the length of the string “Hello, TypeScript!”, which is 12. By using the as
keyword, we ensured that TypeScript treated someValue
as a string, allowing us to perform string operations without errors.
Using the as
Keyword with Interfaces
The as
keyword becomes even more powerful when working with interfaces. Type assertions can help you convert one type into another, especially when dealing with complex data structures. This is particularly common when fetching data from APIs, where the returned data may not match the expected structure.
Consider the following scenario where you fetch user data:
interface User {
name: string;
age: number;
}
const jsonResponse: any = '{"name": "Alice", "age": 30}';
const user: User = JSON.parse(jsonResponse) as User;
In this example, we define a User
interface with name
and age
properties. The jsonResponse
variable holds a JSON string that represents a user. When we parse this JSON string, TypeScript infers its type as any
. By using as User
, we assert that the parsed object conforms to the User
interface.
Output:
{ name: 'Alice', age: 30 }
The output confirms that the parsed object is treated as a User
, allowing us to access its properties confidently. This approach improves type safety and ensures that any operations on the user
object are type-checked against the User
interface.
Type Assertions with Union Types
When working with union types, the as
keyword can be particularly helpful in narrowing down types. Union types allow a variable to hold multiple types, but sometimes you need to specify which type you’re working with for TypeScript to understand your intentions.
Here’s an example:
function processValue(value: string | number) {
if (typeof value === "string") {
console.log((value as string).toUpperCase());
} else {
console.log((value as number).toFixed(2));
}
}
processValue("hello");
processValue(42);
In this code, the processValue
function accepts a parameter that can either be a string or a number. Inside the function, we check the type of value
. If it’s a string, we use as string
to assert its type and call the toUpperCase
method. If it’s a number, we assert it as a number and use toFixed
to format it.
Output:
HELLO
42.00
The output shows that the string was converted to uppercase and the number was formatted to two decimal places. This demonstrates how the as
keyword can help you work with union types effectively, ensuring that your code behaves as expected.
Conclusion
The as
keyword in TypeScript is a powerful tool for type assertions, allowing developers to specify types explicitly and improve type safety in their applications. By using as
, you can work with complex data structures, union types, and interfaces, ensuring that your code is robust and error-free. Mastering the as
keyword will not only enhance your TypeScript skills but also lead to cleaner and more maintainable code. As you continue your journey with TypeScript, remember to leverage the as
keyword to make your code more predictable and reliable.
Frequently Asked Questions
-
What is the purpose of the
as
keyword in TypeScript?
Theas
keyword is used for type assertions, allowing developers to specify a type for a variable, overriding TypeScript’s inferred type. -
Can I use the
as
keyword with any type?
Yes, you can use theas
keyword to assert any type, but it is essential to ensure that the assertion is accurate to avoid runtime errors. -
How does the
as
keyword improve type safety?
By explicitly defining types, theas
keyword helps catch potential type-related errors at compile time, making your code more reliable. -
Are there alternatives to the
as
keyword for type assertions?
Yes, you can also use angle-bracket syntax for type assertions, but it is less common in JSX files, where theas
keyword is preferred. -
When should I avoid using the
as
keyword?
You should avoid usingas
when you are unsure about the type of a variable, as incorrect assertions can lead to runtime errors.