How to Format String in TypeScript
- Appending of Strings to Form New Strings in TypeScript
-
Use
Template
Strings to Form Strings in TypeScript
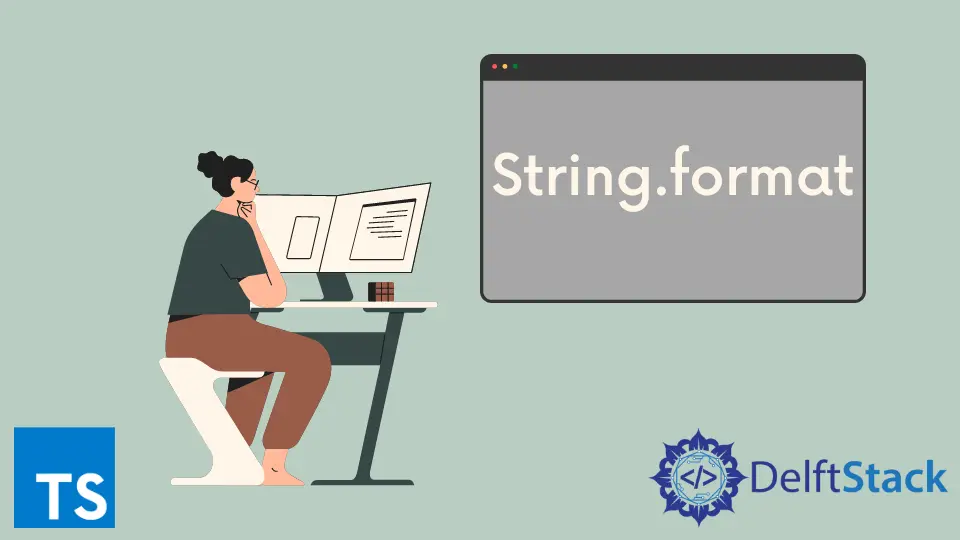
A string is a set of 16-bit unsigned integer values(UTF-16 code units). Strings in TypeScript are immutable, i.e., values at particular indexes cannot be changed, but strings or character values can be appended at the end of strings.
However, characters at particular indexes can be read due to the read-only property of strings.
Appending of Strings to Form New Strings in TypeScript
In TypeScript, strings can be appended to form new strings. The +
or the concatenation operator joins two strings to form the final string.
// string literal
var name : string = 'Geralt';
var nameString = 'The Witcher is of age 95 and his name is ' + name ;
console.log(nameString);
Output:
"The Witcher is of age 95, and his name is Geralt"
However, this becomes inconvenient in the case of large strings, and thus template
strings were introduced in TypeScript.
Use Template
Strings to Form Strings in TypeScript
Template
Strings are string literals allowing embedded expressions. They use string interpolation through the ${``}
operator, and any expression can be thus embedded.
var name : string = "Geralt";
var age : number = 95;
var formedString = `The Witcher is of age ${age} and his name is ${name}`;
console.log(formedString);
Output:
"The Witcher is of age 95, and his name is Geralt"
Note the instead of the general ""
or ''
in case of strings. Thus template strings allow a major cleaner way of representing strings.
Moreover, with template strings, on-the-fly processing becomes very convenient.
var name : string = "Geralt";
var age : number = 95;
var enemiesKilledPerYear = 3;
var formedString = `The Witcher is of age ${age} and his name is ${name} and has killed over ${age * enemiesKilledPerYear} enemies`;
console.log(formedString);
Output:
"The Witcher is of age 95, and his name is Geralt and has killed over 4750 enemies"
Moreover, string templates can generate multiline strings using (``)
.
var formedString = `Today is a nice day
to learn new things about TypeScript`;
console.log(formedString);
Output:
"Today is a nice day
to learn new things about TypeScript"