How to Run File in TypeScript
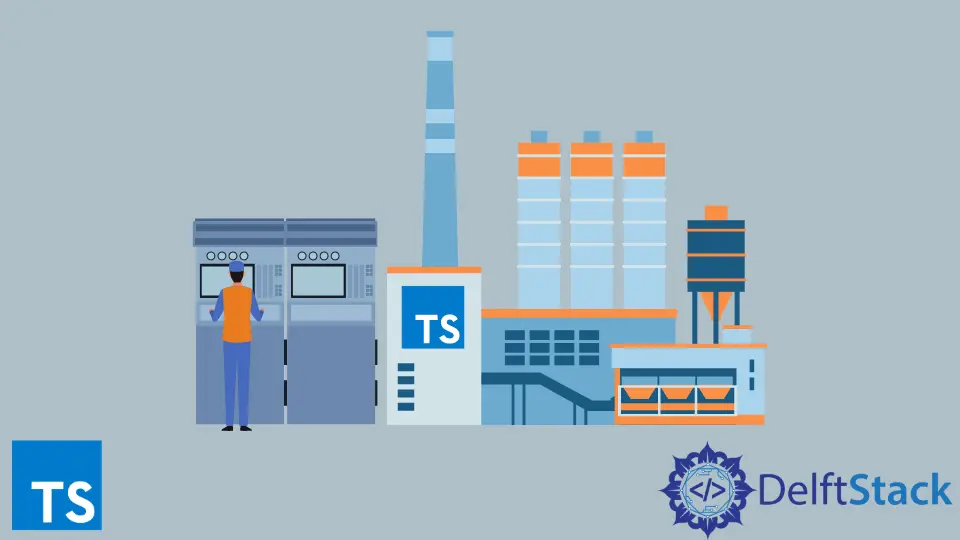
This article will discuss different methods of running files in TypeScript.
Prerequisites to Run File in TypeScript
The first prerequisite for this is Node.js
. We can check if we have installed Node locally or not by using the following command as shown below.
node -v
This command will display the version of Node installed on our device. We can install Node from the Node website.
The next prerequisite is TypeScript
. To execute the TypeScript files, we have to install the TypeScript compiler.
We can run the below command to confirm if we successfully installed it.
tsc -v
It’s assured which TypeScript version we are presentably using on the device. We can install it with npm
or another package manager if not installed.
We can install TypeScript globally by running the following command as shown below.
npm install -g typescript
Run File in TypeScript
If the prerequisites mentioned above are satisfied, we are ready to execute the files of TypeScript by using the command line.
tsc main.ts
node main.js
As shown above, by executing the command, we will generate a js
file with the same name at the runtime in TypeScript. We performed the generated js
file to run node main.js
.
We created some content in the TypeScript file, mars.ts
. To execute, we need to run the below commands.
tsc mars.ts
node mars.js
This command will open the file with the name mars
and displays the result.
Let’s go through another example in which we can utilize the ts-node
module to execute the TypeScript file from the command line.
We can install it with npm
or another module manager with the below command.
npm install -g ts-node
Then we will execute the TypeScript files with the command below.
ts-node main.ts
This command will open the file with main
and display the result.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn