How to Use Jest Mock in TypeScript
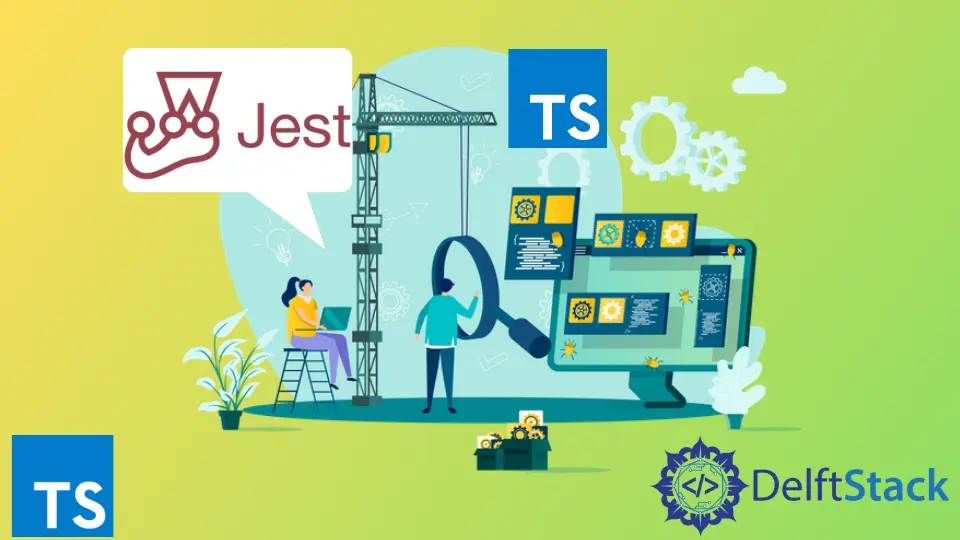
We will introduce Jest Mock
and how to use it in TypeScript with an example.
Jest Mock in TypeScript
When working on commercial applications that need to be perfect for the users, we must ensure that each module works how we want it to work. For this purpose, we use Jest mocks in TypeScript.
Jest mock is a testing framework for a large web application. By using the mock
function, we can spy on the modules of a function called indirectly by some different code instead of only testing the output.
The mock
function will return undefined
when invoked if no implementation is given. We can easily use the jest.mock()
function as shown below.
import * as dependency from '../someModule';
jest.mock('../someModule');
The jest.mock()
function will change the type of the dependency, because of which we need to use type casting after calling the jest.mock()
function in TypeScript. We can easily call the type casting by using the typeof
method in TypeScript.
Let’s go through an example in which we will use an automatic mock to test the module we import into TypeScript, as shown below.
import { testClass } from './testClass';
jest.mock('./testClass');
const mockClass = <jest.Mock<testClass>>testClass;
But if we want to mock the module or class manually, we can also do it easily. Let’s go through an example in which we will mock the class manually, as shown below.
import testClass from './testClass';
import testClassDependency from './testClassDependency';
const testMockClassDependency = jest.fn<testClassDependency>(() => ({
}));
it('Throw an error', () => {
const testClass1 = new testClass(testMockClassDependency());
});
As we can see from the above example, the testMockClassDependency
will create a mocked object instance. We can use class, type, or an interface instead of testClassDependency
.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn