How to Import JavaScript in TypeScript
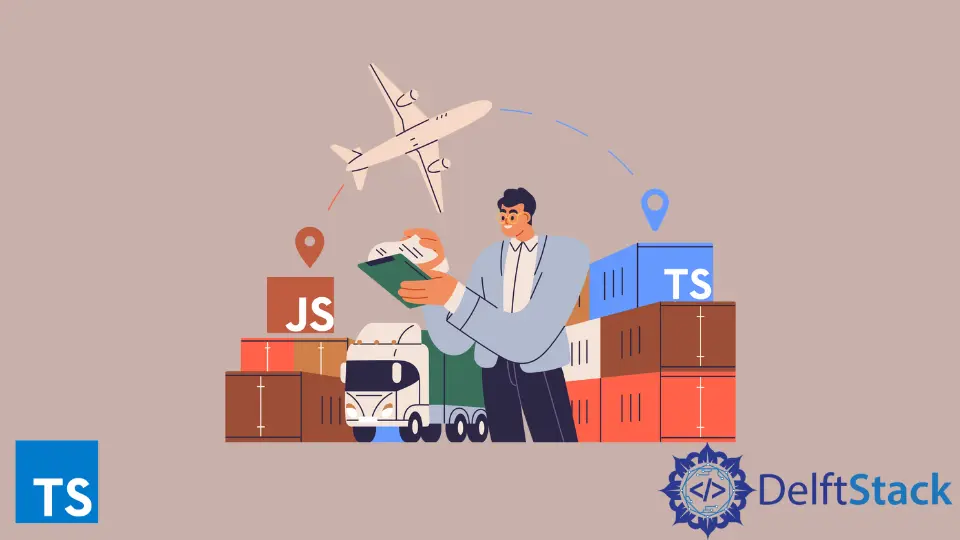
We will discuss how to import JavaScript into the TypeScript language.
Import JavaScript in TypeScript Languages
Before discussing the main topic, we will give a piece of short information about JavaScript and TypeScript programming languages. TypeScript is a high-quality programming language mainly developed and maintained by Microsoft tech company.
The primary purpose of TypeScript is that it is made as a syntactical superset of JavaScript, which has an open-source open compiler and by a single vendor by Microsoft. Transcript catches the type system mistakes, which makes JavaScript more efficient.
JavaScript is most famous as a web language. Website made by JavaScript gives an interactive view to the users, which helps engage them. Some advantages of using JavaScript are Server load, rich interface, and versatility.
Importing JavaScript in TypeScript is a simple method. Now we start the process of converting JavaScript into TypeScript.
If we are working on JavaScript, we should run our JavaScript directly. We are working on a .js
file where we are in the dictionary like src
, lib
, and dist
, then run as a desired.
Suppose we are writing in JavaScript and want to add our written data as input into TypeScript in this condition. In that case, we need to separate your input and output files to prevent TypeScript from overwriting them.
The allowJs
setting permits JavaScript files to be imported inside our TypeScript files. The setting essentially allows JavaScript and TypeScript files to live in identical projects.
Let’s have an example file that we need to import into TypeScript, which has path ./js/Programming_file.js
. In this first method, we use the require()
function, we can import files, as shown below:
# typescript
var Programming_file = require('./../js/programming_file');
In this second method, we import the JavaScript file into the typescript using the import {var_name}
function.
# typescript
import {Programming_file} from './../js/Programming_file'
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn