The console.log Method in TypeScript
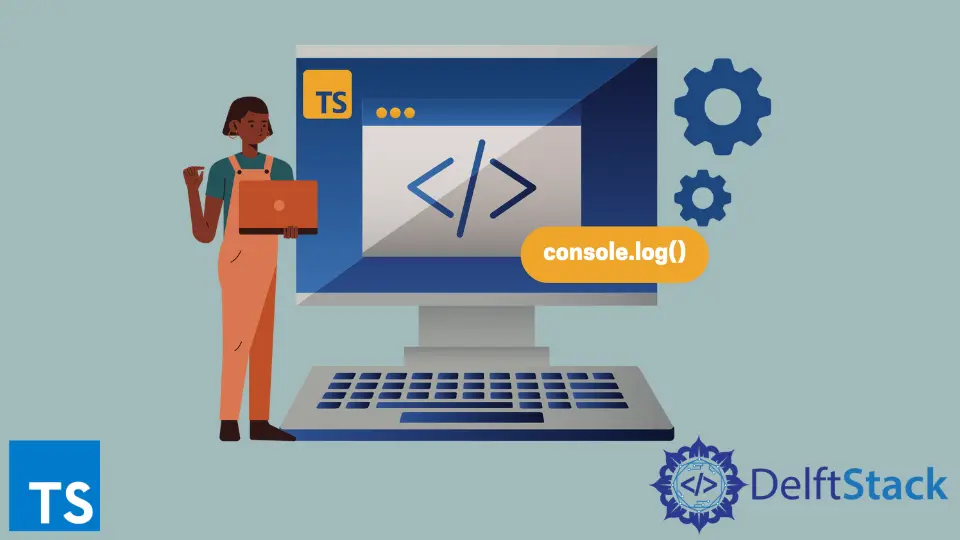
This article will introduce console.log()
in TypeScript and how it works in TypeScript with examples. We will discuss different segments of it.
Use the console.log()
Method in TypeScript
In the console.log()
method, we can output a message to the web console. The message may be a single string or multiple JavaScript objects.
Here is an example of console.log()
in TypeScript. This feature must be available only in Web Workers.
Code:
# Typescript
let name = "Hasnain"
let id = "Bc140402269"
let num = 13
let msg = "Hello"
console.log(name)
console.log(id)
console.log(num)
console.log(msg)
Output:
A list of JavaScript is an object to the output. The representations of a string of each object are appended together to work and listed.
Be Aware if we log things using the new versions of browsers such as Chrome and Firefox, we will get the result in the console as a reference to the object, which is not the "value"
of the thing at the same time when we call console.log()
. Still, we will get the value of the object at the time when we open the console.
A JavaScript string has zero or more substitution strings. We can replace substitution strings within msg in JavaScript objects, giving us additional control over the output format.
We will discuss console.log()
with examples in which we will try to log a string and a number. Let’s try console.log()
with a string variable.
Code:
# Typescript
let hello = 'hello world'
console.log(hello)
Output:
Now, let’s try console.log()
with a number.
Code:
# Typescript
let evenNumber = 2
console.log('the even is')
console.log(evenNumber)
Output:
As the above examples demonstrate, we can use console.log()
for any message in our console.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn