How to Cast a Number to String in TypeScript
- Method 1: Using the String() Function
- Method 2: Using the toString() Method
- Method 3: Using Template Literals
- Method 4: Using String Concatenation
- Conclusion
- FAQ
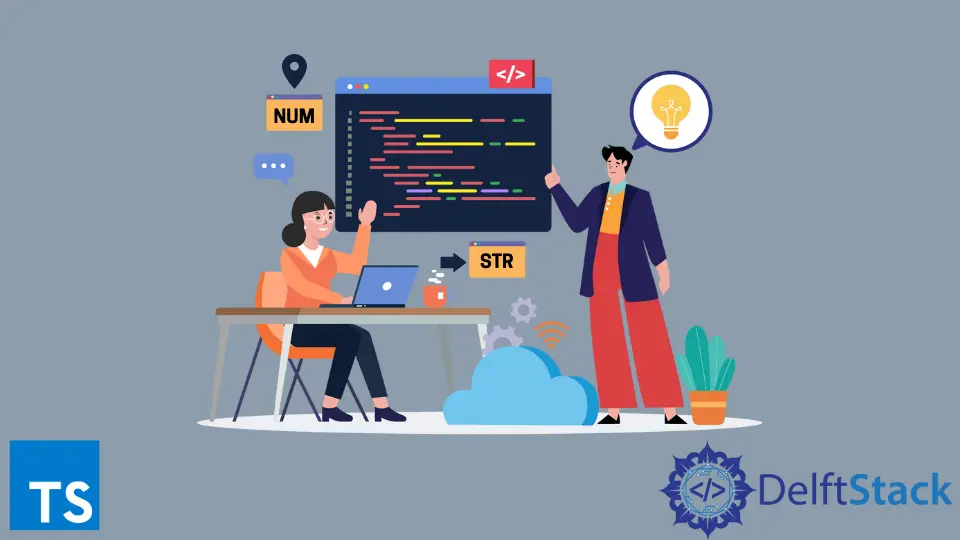
Casting a number to a string in TypeScript is a fundamental skill that every developer should master. Whether you’re preparing data for display, formatting outputs, or simply manipulating strings, knowing how to effectively convert numbers to strings can streamline your coding process.
In this tutorial, we’ll explore various methods to achieve this conversion, including some practical examples. By the end of this guide, you will have a solid understanding of how to cast numbers to strings in TypeScript, enabling you to handle data more efficiently. Let’s dive in and discover these techniques together!
Method 1: Using the String() Function
One of the most straightforward ways to convert a number to a string in TypeScript is by using the built-in String()
function. This method is simple and effective, making it a popular choice among developers. When you pass a number to the String()
function, it returns the number as a string.
Here’s how you can use this method in TypeScript:
let num: number = 123;
let str: string = String(num);
console.log(str);
Output:
123
In this example, we declare a variable num
of type number
and assign it the value 123
. By passing num
to the String()
function, we convert it into a string and assign it to the variable str
. The result is then logged to the console, displaying the string representation of the number. This method is particularly useful when you want to ensure that the conversion is done in a clear and readable way.
Method 2: Using the toString() Method
Another effective way to cast a number to a string in TypeScript is by using the toString()
method. This method is available on all number objects and is specifically designed for converting numbers to their string representation. It provides a more object-oriented approach to the conversion process.
Here’s an example of how to use the toString()
method:
let num: number = 456;
let str: string = num.toString();
console.log(str);
Output:
456
In this snippet, we again declare a variable num
with the value 456
. By calling the toString()
method on num
, we convert it into a string and store it in the variable str
. The output is then printed to the console. This method is particularly advantageous when you are working with number instances and want to maintain a clean and concise code structure.
Method 3: Using Template Literals
Template literals in TypeScript provide a modern and versatile way to create strings, including converting numbers to strings. By embedding expressions within backticks, you can easily convert numbers while simultaneously formatting them as needed. This method is not only effective but also enhances readability.
Here’s how you can use template literals for this conversion:
let num: number = 789;
let str: string = `${num}`;
console.log(str);
Output:
789
In this example, we declare a variable num
and assign it the value 789
. By enclosing num
in backticks and using the ${}
syntax, we create a string that includes the number. The string is then logged to the console. Template literals are particularly useful when you need to concatenate multiple values or format strings in a more complex manner.
Method 4: Using String Concatenation
String concatenation is another simple yet effective method to convert a number to a string in TypeScript. By concatenating a number with an empty string, you can force the conversion to occur. This method is quick and can be particularly handy in various situations.
Here’s an example of how to use string concatenation:
let num: number = 101112;
let str: string = num + '';
console.log(str);
Output:
101112
In this code, we declare a variable num
with the value 101112
. By adding an empty string (''
) to num
, we implicitly convert it to a string and store the result in str
. The output is then displayed in the console. This method is especially useful for quick conversions when you do not need to worry about readability or clarity.
Conclusion
In this tutorial, we explored several methods for casting a number to a string in TypeScript. From using the String()
function and the toString()
method to leveraging template literals and string concatenation, each approach has its own advantages. Depending on your specific use case, you can choose the method that best fits your coding style and needs. Mastering these techniques will not only enhance your TypeScript skills but also improve your overall efficiency as a developer. Happy coding!
FAQ
-
What is TypeScript?
TypeScript is a superset of JavaScript that adds static types, allowing for better tooling and error-checking during development. -
Why would I need to cast a number to a string?
Casting numbers to strings is often necessary for formatting outputs, concatenating with other strings, or preparing data for display. -
Can I use any of these methods in JavaScript?
Yes, all the methods discussed in this article are applicable in JavaScript as well. -
Is there a performance difference between these methods?
Generally, the performance difference is negligible for most applications, but usingString()
andtoString()
may be slightly faster in some cases. -
Are there any edge cases to consider when converting numbers to strings?
Yes, be cautious with special values likeNaN
,Infinity
, or-Infinity
, as their string representations may differ from standard numbers.
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn