How to Add TypeScript to a React Project
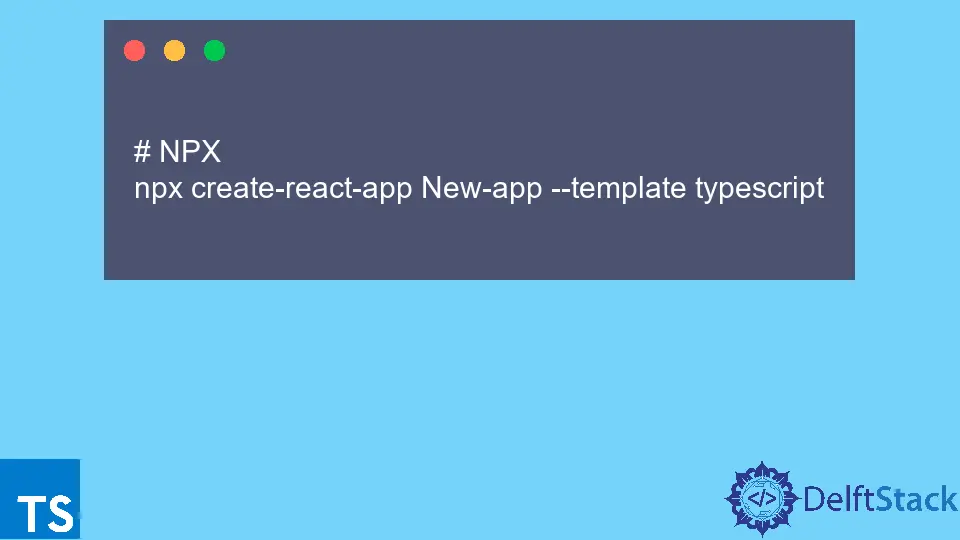
We will introduce how to add TypeScript to new and old react projects using simple commands.
Add TypeScript to a React Project
Many developers prefer to use TypeScript in both React and Angular projects. Sometimes we get applications or projects built in JavaScript and want to add TypeScript to these projects.
In this tutorial, we’ll go through both scenarios in which we create a new React application or add a TypeScript to a project already built. First, let’s start with the new installation of react application.
There are two ways to install React application using the TypeScript template. One is by using npx
.
Using the following command, we can easily install a new React application with the TypeScript template.
# NPX
npx create-react-app New-app --template typescript
This command will install a new react application with the latest version. Now, if we want to install the react application using the yarn
, we can run the below command.
# YARN
yarn create-react-app New-app --template typescript
Now, let’s go through our second scenario in which we already have a project built using the JavaScript template and want to convert it into a TypeScript framework.
First, we need to run the following command to install TypeScript into our project using npm
, as shown below.
# NPM
npm install --save typescript @types/node @types/react @types/react-dom @types/jest
If we have installed our project using yarn
, we need to run the command below.
# YARN
yarn add typescript @types/node @types/react @types/react-dom @types/jest
Once we have installed the TypeScript and its dependencies, we can rename the JavaScript files from .js
to .ts
. After changing all the files to TypeScript, we will restart our development server.
There will be some errors related to types. We must fix these errors according to the project’s needs before developing new features.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn