The abstract Method in TypeScript
- What is an Abstract Method?
- Defining an Abstract Method in TypeScript
- Benefits of Using Abstract Methods
- Best Practices for Using Abstract Methods
- Conclusion
- FAQ
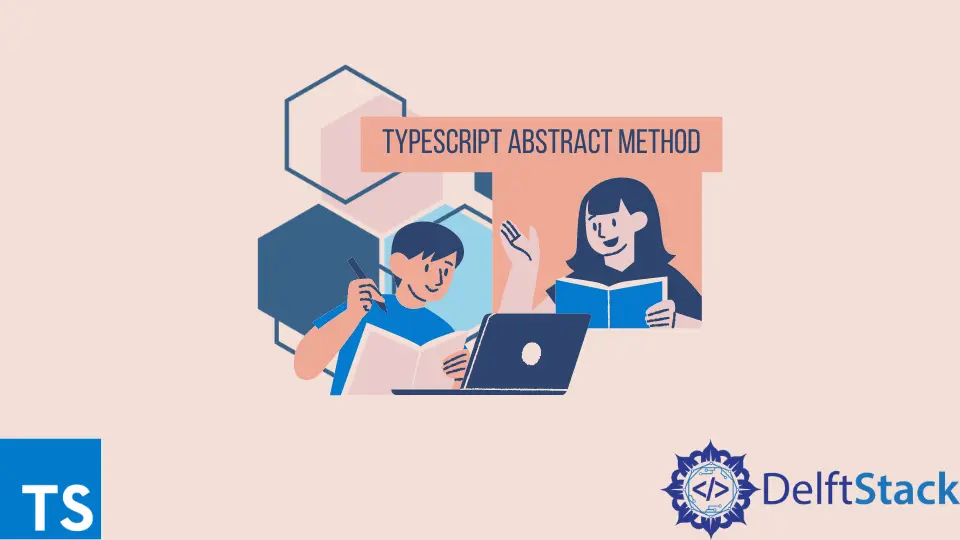
TypeScript, a superset of JavaScript, enhances the language with static typing and powerful features that improve code quality and maintainability. One such feature is the abstract method, which plays a crucial role in object-oriented programming.
In this article, we will explore what an abstract method is, how to define it, and its significance in TypeScript. By the end, you’ll have a solid understanding of abstract methods, enabling you to write cleaner and more efficient code. So, let’s dive into the world of TypeScript and uncover the potential of abstract methods!
What is an Abstract Method?
An abstract method is a method that is declared in an abstract class but does not have an implementation. This means that any subclass that inherits from the abstract class must provide its own implementation of the abstract method. Abstract methods serve as a blueprint for subclasses, ensuring that they adhere to a specific interface while allowing for flexibility in implementation.
In TypeScript, you can create an abstract class using the abstract
keyword. This class can contain both abstract methods and concrete methods (methods with implementations). The primary purpose of abstract methods is to enforce a contract for subclasses, making it clear that they must implement specific functionality.
Defining an Abstract Method in TypeScript
To define an abstract method in TypeScript, follow these steps:
- Create an abstract class using the
abstract
keyword. - Declare the abstract method within the class using the
abstract
keyword. - Implement the abstract method in any subclass that extends the abstract class.
Here’s a simple example to illustrate this:
abstract class Animal {
abstract makeSound(): void;
move(): void {
console.log("The animal moves");
}
}
class Dog extends Animal {
makeSound(): void {
console.log("Woof! Woof!");
}
}
const dog = new Dog();
dog.makeSound();
dog.move();
Output:
Woof! Woof!
The animal moves
In this example, we define an abstract class called Animal
, which includes an abstract method makeSound
. The Dog
class extends Animal
and provides its own implementation of the makeSound
method. When we create an instance of Dog
and call both methods, we see the expected output. This structure enforces that any subclass of Animal
must implement the makeSound
method, promoting consistency across different animal types.
Benefits of Using Abstract Methods
Using abstract methods in TypeScript offers several benefits:
-
Code Reusability: Abstract classes allow you to define shared functionality that can be reused across multiple subclasses. This reduces code duplication and promotes cleaner code.
-
Enforcement of Contracts: Abstract methods ensure that all subclasses adhere to a specific interface. This guarantees that certain methods will be implemented, leading to more predictable and reliable code.
-
Improved Maintainability: By defining a clear structure through abstract classes and methods, your code becomes easier to maintain. Changes can be made in the abstract class, and all subclasses will inherit those changes.
-
Enhanced Readability: Abstract methods provide a clear indication of what functionality is expected in subclasses. This improves code readability and helps other developers understand the intended structure of your code.
Best Practices for Using Abstract Methods
When working with abstract methods in TypeScript, consider the following best practices:
-
Use Abstract Classes Wisely: Only use abstract classes when you have a clear hierarchy of classes that share common functionality. Avoid overusing abstract classes, as they can add unnecessary complexity.
-
Keep Methods Focused: Each abstract method should have a single responsibility. This aligns with the single responsibility principle and makes your code easier to understand and maintain.
-
Document Your Code: Provide clear documentation for your abstract methods. This helps other developers understand the expected behavior and implementation details.
-
Leverage TypeScript Features: Take advantage of TypeScript’s type system to define method parameters and return types in your abstract methods. This enhances type safety and reduces potential runtime errors.
Conclusion
Understanding abstract methods in TypeScript is vital for anyone looking to write clean, maintainable, and efficient code. By leveraging abstract classes and methods, you can enforce a contract for your subclasses while promoting code reusability and readability. As you continue to explore TypeScript, keep these concepts in mind to elevate your programming skills. Happy coding!
FAQ
-
What is the purpose of an abstract method in TypeScript?
An abstract method serves as a blueprint in an abstract class, enforcing that subclasses must provide their own implementation. -
Can an abstract class have concrete methods?
Yes, an abstract class can contain both abstract and concrete methods. -
How do I implement an abstract method in a subclass?
You simply extend the abstract class and provide an implementation for the abstract method in the subclass.
-
Is it possible to instantiate an abstract class?
No, you cannot create an instance of an abstract class directly. You must create an instance of a subclass that implements the abstract methods. -
What are the advantages of using abstract classes?
Abstract classes promote code reusability, enforce contracts for subclasses, improve maintainability, and enhance code readability.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn