How to Print the Value of the Tensor Object in TensorFlow
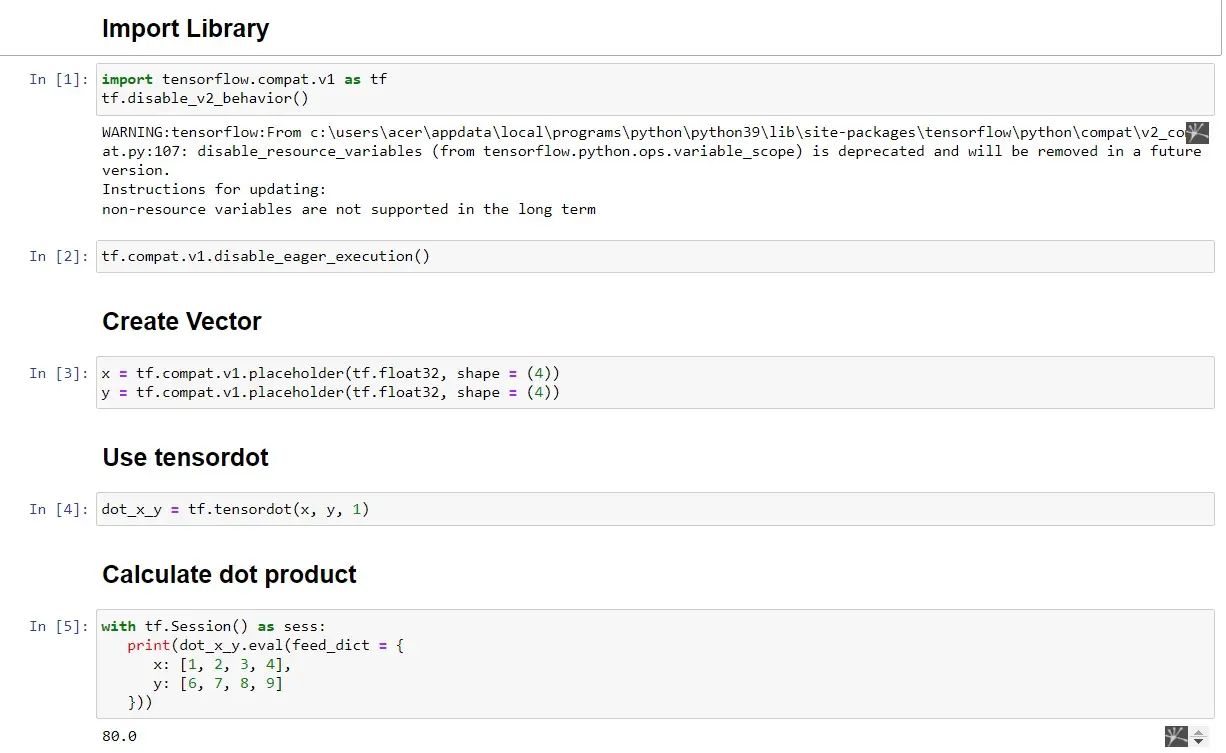
In this post, we will print the value of the tensor object in TensorFlow.
Print the Value of the Tensor Object in TensorFlow
Google created the open-source library TensorFlow to execute deep-learning neural networks and machine-learning models in a browser or node environment. tf.print
is used here to print the information and data of tf.Tensor
.
A tensor is an n-dimensional vector or matrix that may represent any data. A tensor’s values all have the same data type and known (or at least partially known) form.
A tensor might start as input data or as calculation output. In machine learning, feature vectors are items used to feed models.
Any data can be a feature vector. Usually, the feature vector serves as the main input while populating a tensor.
To create a tensor, we will use tf.constant
here. Let’s see an example.
First, import the required library:
Now let’s create an object of tensor. For that, we have used tf.constant
.
See the screenshot below:
Let’s perform some action on the tensor object. We have added the value of the tensor object:
Let’s print the value of the tensor object using tf.print
.
In this way, we can create a tensor object and print it.
Screenshot of the full code:
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn