TensorFlow Feed_dict
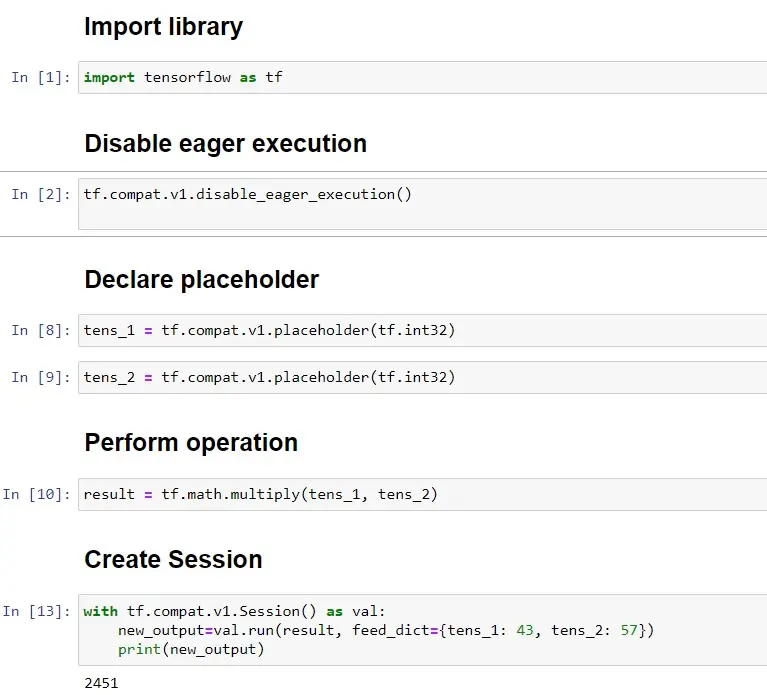
Let’s understand TensorFlow feed_dict
in this article.
Use feed_dict
in TensorFlow
We need placeholders
and feed dictionaries
to be able to run the same model on multiple problem sets. Our visualization must keep up with the complexity of our TensorFlow application.
TensorFlow placeholders are the same as variables anybody may specify, even during runtime, using the feed_dict
parameter. TensorFlow uses the feed_dict
option to feed values to these placeholders to avoid an error that requests you to feed a value for placeholders in TensorFlow.
TensorFlow placeholders are comparable to variables and may be declared using tf.placeholder
.
You do not need to supply an initial value. You may specify it at runtime using the feed_dict
parameter within Session.run
, whereas tf.Variable
requires an initial value when declared.
Every session includes fetches
and feed_dict
. The fetches
argument indicates what we want to compute, whereas the feed dictionary offers placeholder values for that computation.
Let’s look at the syntax and how the tf.compat.v1.placeholder()
method in Python TensorFlow works.
Syntax:
tf.compat.v1.placeholder(dtype, shape=None, name=None)
Parameters:
- The
dtype
option indicates the kind of elements in the tensor. Shape
accepts no value by default, and if you don’t specify a shape in the tensor, you can feed any tensor.Name
is an optional argument that gives the operation’s name.
Let’s look at an example of using TensorFlow to generate a feed_dict
in placeholder
.
First, import the library which is required for the task.
Then create a disable_eager_execution
that will handle the placeholder
conflict with TensorFlow versions.
After that, declare a placeholder
of the int32
data type.
Then perform actions on the placeholder
. For that, we have used the multiply function tf.math.multiply
.
We assigned the feed_dict
as an argument when we created the session. The placeholder
values are provided via feed_dict
.
Here is the screenshot of the full code.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn