How to Calculate Dot Product in TensorFlow
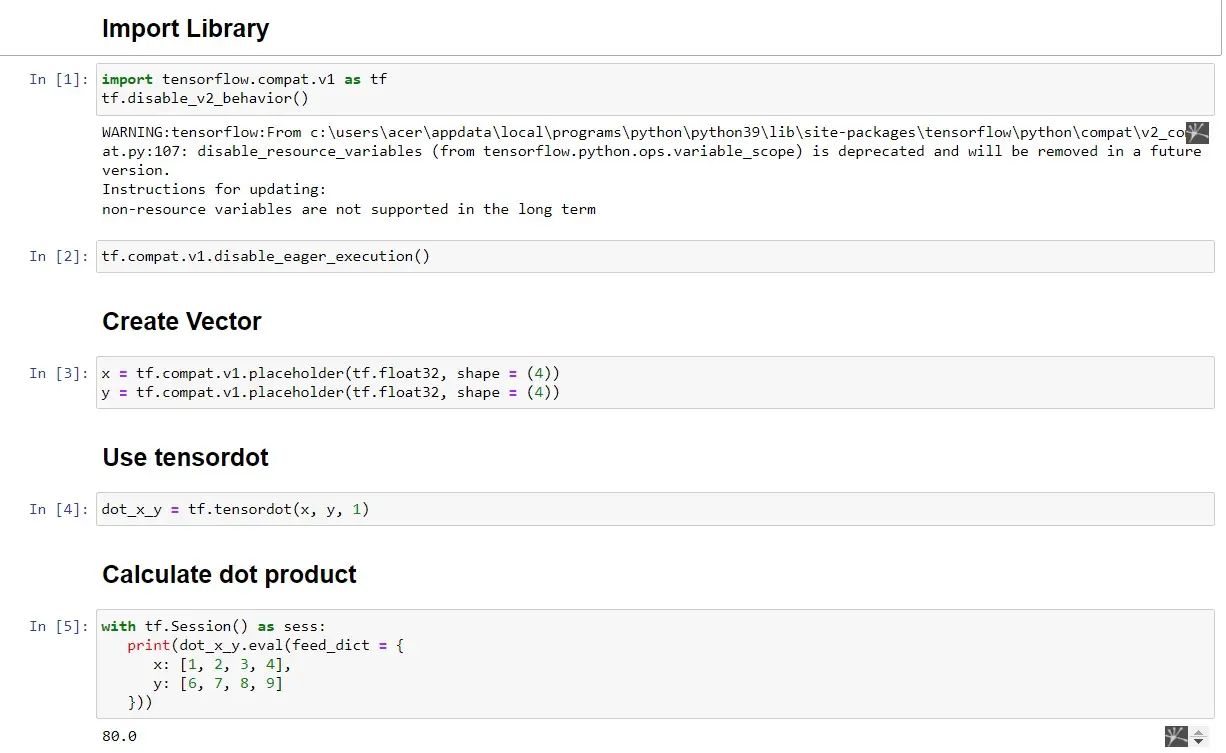
In this post, we will learn how to calculate the dot product of two vectors in TensorFlow.
TensorFlow Dot Product
To calculate the dot product, we will use the tensordot
of TensorFlow.
Tensordot
is a fantastic tool for tensor operations that may be used in optimization, engineering, machine learning, and any place else multidimensional operations are required. Tensordot
sums the product of two (x and y) elements over the indices provided by the axis.
Tensordot seems to stick after you completely comprehend it. If you’ve worked with matrices before, the operation isn’t mathematically rigorous; rather, it’s a visual/perceptual enlargement of what you already know.
Let’s take a step back from the matrix dot product and start from the beginning with tensordot
with vectors.
Tensordot
with vectors can help you develop a strong intuition. It follows the same patterns as a matrix dot product; the only difference is that we will examine the dot product along the chosen axes.
First, import the necessary libraries required.
It will import a TensorFlow as tf
, which allows writing code that works in both TensorFlow version(1 & 2), and disable_eager_execution
helps to solve the run-time errors if it occurs.
Now create a vector that holds the data for calculation. For that, we will be using placeholder
.
The placeholder
always fed with three parameters, i.e. Datatype=tf.float32
, shape = 4
.
Now use tensordot
for the multiplication of two vectors.
Tensordot
takes three parameters, i.e., vector x
, y
, and axes
.
We will experiment with integer input types to examine the axis parameter inputs thoroughly. The integer input simplifies typical examples of dot products, and both are connected.
When the axes
parameter is set to 1, the dot product is performed for the whole instance along the 0 axes for x and 0 axes for y (multiply, then add). The unusual language would be as follows: take the final axis of x and do the dot product with the last axis of y.
A session enables the execution of graphs or parts of graphs. It allocates resources (on one or more computers) and stores the real values of intermediate outcomes and variables.
This will give the sum after the product of two vectors.
This is a typical dot product frequently used in schools and early matrix algebra.
Full code:
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn