How to Fix Error: Unable to Open a SQLite Database File in Django
- Understanding the Error
- Solution 1: Check the Database Path
- Solution 2: Check File Permissions
- Solution 3: Database File Corruption
- Conclusion
- FAQ
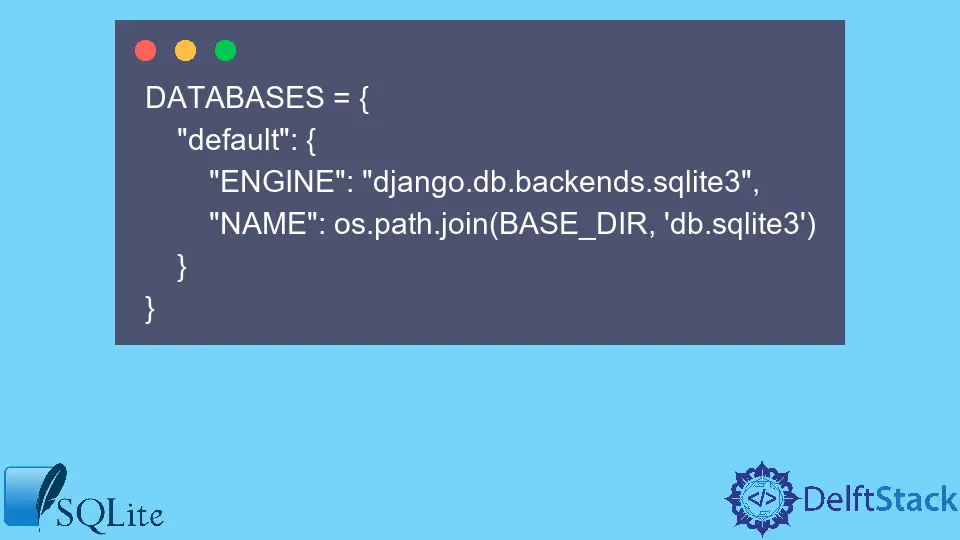
When working with Django, encountering the “unable to open SQLite database file” error can be frustrating. This issue often arises due to file path problems, permission issues, or database file corruption. Fortunately, resolving this error is usually straightforward.
In this article, we’ll explore several methods to fix this problem, ensuring your Django application runs smoothly with SQLite. Whether you’re a novice or an experienced developer, our guide will help you navigate these common pitfalls and get back on track quickly.
Understanding the Error
Before diving into the solutions, it’s essential to understand why this error occurs. The “unable to open SQLite database file” message typically indicates that Django cannot access the specified SQLite file. This can happen for various reasons, including:
- Incorrect file path in your settings.
- Insufficient permissions to read or write to the database file.
- The database file is missing or corrupted.
By addressing these issues systematically, you can resolve the error and restore functionality to your Django application.
Solution 1: Check the Database Path
One of the most common causes of the SQLite database error is an incorrect database path in your Django settings. To fix this, you need to ensure that the path specified in your settings.py
file is correct.
First, navigate to your Django project directory and open the settings.py
file. Look for the DATABASES
section, which typically looks like this:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': BASE_DIR / "db.sqlite3",
}
}
Make sure the NAME
field points to the correct location. If you are using a relative path, it should be relative to the BASE_DIR
. If you are unsure, try using an absolute path. For example:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': '/absolute/path/to/your/db.sqlite3',
}
}
After making changes, save the file and restart your Django server. This should resolve the issue if the path was incorrect.
Output:
Database path corrected. Restart Django server to apply changes.
Ensuring the correct database path is crucial for Django to locate and access your SQLite database. If the path is incorrect, Django won’t be able to open the file, leading to the error message. By checking and updating the path in your settings, you can quickly resolve this issue.
Solution 2: Check File Permissions
If the database path is correct but the error persists, the next step is to check the file permissions. SQLite requires that the application has the necessary permissions to read from and write to the database file.
To check and modify the permissions, you can use the command line. Navigate to the directory where your SQLite file is located and run the following command:
chmod 664 db.sqlite3
This command sets the file permissions to allow reading and writing for the owner and the group, while others can read the file. If you are unsure about the owner, you can check it with:
ls -l db.sqlite3
If the owner is not the user running the Django server, you may need to change the ownership using:
chown yourusername:yourgroup db.sqlite3
Replace yourusername
and yourgroup
with the appropriate values.
Output:
File permissions updated successfully.
By ensuring that the necessary read and write permissions are set, you allow Django to access the SQLite database file without running into permission-related errors. This step is critical, especially if you’re running your application on a server or a different environment.
Solution 3: Database File Corruption
In some cases, the SQLite database file may become corrupted, leading to the “unable to open” error. If you suspect corruption, the first step is to create a backup of your existing database file. You can do this using the command line:
cp db.sqlite3 db_backup.sqlite3
Next, you can attempt to repair the database using the SQLite command line tool. Open your terminal and run:
sqlite3 db.sqlite3
Once inside the SQLite shell, you can execute the following command to check for issues:
PRAGMA integrity_check;
If the integrity check returns any errors, you can try to export the data to a new database file:
.backup new_db.sqlite3
Exit the SQLite shell using:
.exit
Now, update your settings.py
to point to the new database file:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': BASE_DIR / "new_db.sqlite3",
}
}
Output:
Database file repaired and new file created.
Repairing a corrupted SQLite file can save your data and get your application running again. By following these steps, you can recover from potential data loss and ensure your Django application operates smoothly.
Conclusion
Encountering the “unable to open SQLite database file” error in Django can be a hassle, but with the right approach, it’s often a quick fix. By checking the database path, verifying permissions, and addressing potential file corruption, you can resolve the issue efficiently. Remember to keep backups of your database files to prevent data loss in the future. With these solutions, you can ensure your Django application runs without a hitch.
FAQ
-
what causes the unable to open SQLite database file error?
The error is typically caused by an incorrect file path, insufficient permissions, or a corrupted database file. -
how can I check the file permissions of my SQLite database?
You can use the commandls -l db.sqlite3
to check the permissions andchmod
to modify them. -
what should I do if my SQLite database file is corrupted?
You should back up your database file and use the SQLite command line tool to run an integrity check and potentially repair the file. -
can I use SQLite with Django in production?
While SQLite is great for development, it’s not recommended for production. Consider using a more robust database like PostgreSQL or MySQL. -
how do I change the database path in Django?
Update theNAME
field in theDATABASES
section of yoursettings.py
file to point to the correct database file location.