How to Convert SQLite to MySQL
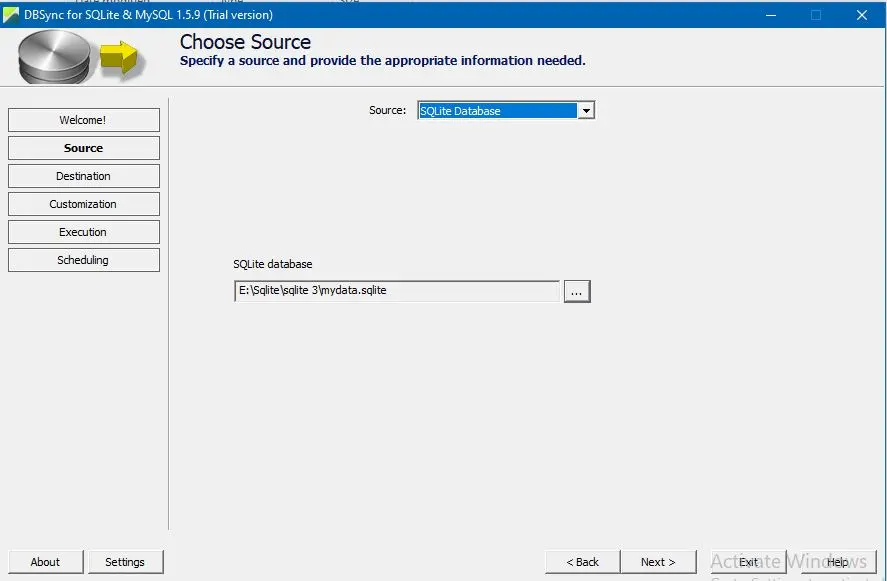
Sometimes we need to convert our database from SQLite to MySQL for various purposes like using some extra features. This article will show the easiest and most simple way to convert an SQLite database into a MySQL database.
Convert SQLite to MySQL
For this purpose, we will use a third-party software named DBConvert. You can download it from here.
Follow the below steps to convert SQLite to MySQL.
-
Open the DBConvert software.
-
In the
"Source"
select theSQLite database
and browse the source of the SQLite file.Now confirm the tables.
Click on
"Next"
. -
In the Destination, select
"MySQL"
and provide all necessary server information. Click on"Next"
. -
Lastly, finish the Customization, Execution, and Scheduling with the necessary information and complete the conversion.
An Alternative Way to Convert SQLite to MySQL
Another easiest alternative way is to use the .dump
command. This command can be used for migrating from SQLite to MySQL.
To convert SQLite to MySQL, use the following command:
sqlite3 mydata.sqlite .dump > mydata.sql
After executing the above command, you will see a .sql
file created in your working directory.
Note that this article is for switching SQLite to MySQL.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn