Stored Procedures in SQLite
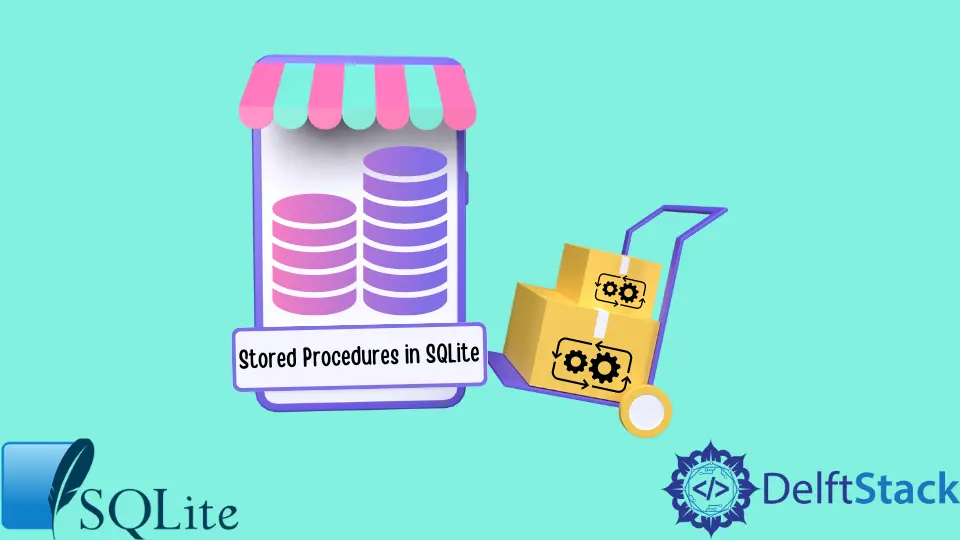
A DBMS or Database Management System is software that enables us to manage a database by performing various CRUD (Create
, Read
, Update
, and Delete
) operations, configuring security, assigning roles, and defining custom access. A DBMS manages various complexities efficiently, such as concurrent access, role-based access, security, and efficient and fast retrieval.
Stored procedures refer to SQL code that is stored on a database and can be reused or executed over and over whenever needed. A stored procedure is like a regular function in most programming languages.
Most database systems nowadays, such as MySQL and PostgreSQL, support stored procedures because they offer scalability and ease of overall database management. In this article, we will talk about stored procedures in the SQLite database.
Stored Procedures in SQLite
Unfortunately, an SQLite database doesn’t offer stored procedures. It is a lightweight database that is not meant for production applications, and hence, various common DBMS features such as high concurrency, robust and precise access control, built-in methods, and stored procedures are missing from this database.
However, suppose we’re using a SQL interface to control an SQLite database from some programming language such as Python, JavaScript, Perl, etc. In that case, we can create our custom user-defined methods and use these methods with regular SQL statements.
This is just a way of mimicking stored procedures’ functionality and features. This way, the reusable code will not exist on the database but in the host programming language.
Additionally, suppose we’re using an ORM or Object Relational Mapping such as Django ORM. In that case, we can define our custom methods inside models’ or managers’ definitions to run over QuerySets
that will return the required output.