How to Insert Multiple Rows in SQLite
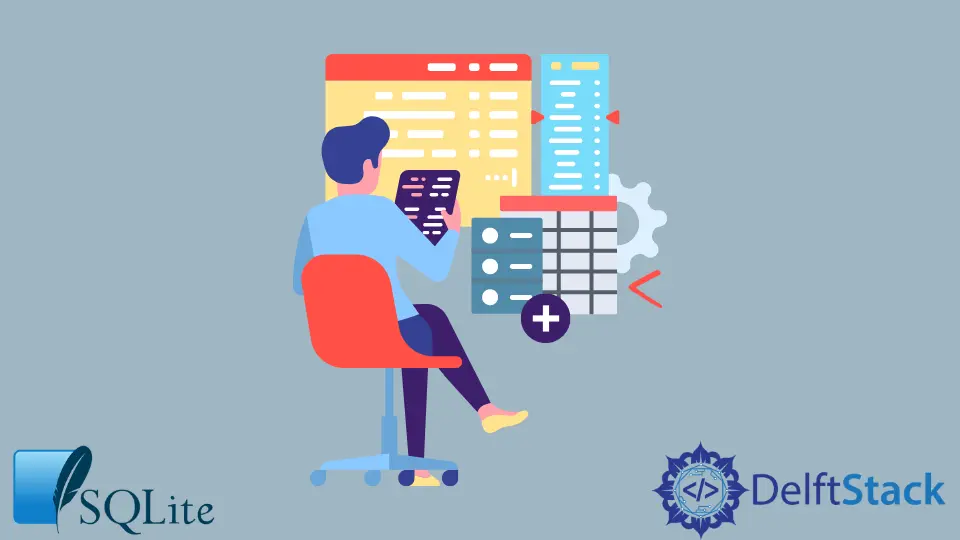
If you are working with any query language, you may work with INSERT
. This keyword is used to insert data into the database.
When you are working with a large number of data, inserting data one by one is very slow.
But there is a way through which we can insert multiple data at a time. For this purpose, we are going to use SQLite.
This article will show us how we can insert multiple data at a time in SQLite, and we will see an example with an explanation to make the topic easier.
The general syntax for the example we are going to use is as follows:
INSERT INTO tablename VALUES
('data', 'data', 'data'),
('data', 'data', 'data');
Insert Multiple Values at a Time in SQLite
In our example below, we will demonstrate how we can insert multiple data at a time using SQLite. Take a look at the below example query.
INSERT INTO demo VALUES
(27, 'Ales', 'null'),
(28, 'Boris', 'null');
In the query above, we inserted two sets of data (27, 'Ales', 'null')
and (28, 'Boris', 'null')
into the table demo
.
After executing the above command, you will get an output like the one below.
This way, we can insert multiple rows in our database using a single line of command, and we don’t need to use a query for each insertion. Also, this is a time-efficient method of inserting multiple data into the database.
Please note that to use this feature, you must install the latest version of SQLite. Some of the backdated version doesn’t allow this feature.
The queries we provided in this article are written for SQLite.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn