How to Get Column Names in SQLite Database
- Method 1: Using the PRAGMA Statement
- Method 2: Querying the SQLite Master Table
- Method 3: Using the SQLite Command Line Interface
- Conclusion
- FAQ
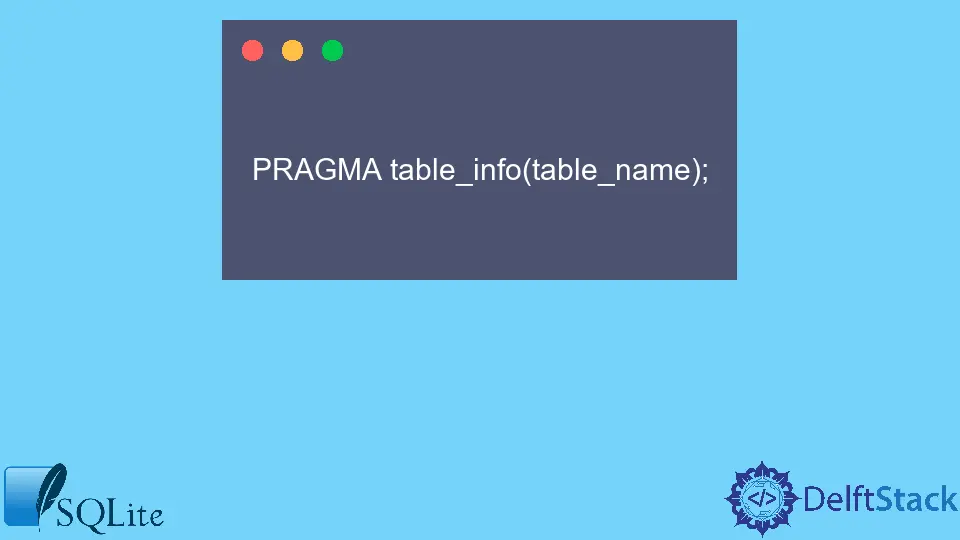
When working with SQLite databases, one common task is retrieving the column names from a specific table. Whether you’re a seasoned developer or just starting out, knowing how to access this information is crucial for effective database management.
In this article, we’ll explore various methods to retrieve column names in SQLite, focusing on practical approaches that you can implement in your projects. By the end, you’ll have a solid understanding of how to navigate SQLite databases and extract the information you need. Let’s dive in!
Method 1: Using the PRAGMA Statement
SQLite provides a handy command called PRAGMA table_info()
that allows you to retrieve details about the columns in a specific table. This command returns a result set that includes the column names, data types, and other attributes. Here’s how you can use it in Python.
import sqlite3
connection = sqlite3.connect('example.db')
cursor = connection.cursor()
cursor.execute("PRAGMA table_info(your_table_name);")
columns_info = cursor.fetchall()
column_names = [column[1] for column in columns_info]
print("Column Names:", column_names)
connection.close()
Output:
Column Names: ['id', 'name', 'age', 'email']
The code starts by establishing a connection to the SQLite database named example.db
. After creating a cursor object, it executes the PRAGMA table_info(your_table_name)
command, which retrieves information about the specified table. The fetchall()
method collects all the rows returned by the query. We then extract the column names using a list comprehension that focuses on the second element of each tuple in the result set. Finally, we print the list of column names and close the connection. This method is straightforward and efficient for obtaining column names.
Method 2: Querying the SQLite Master Table
Another effective way to get column names is by querying the sqlite_master
table, which stores information about all tables and their structures in the database. This method can be particularly useful when you want to retrieve column names dynamically. Here’s how you can do it in Python.
import sqlite3
connection = sqlite3.connect('example.db')
cursor = connection.cursor()
cursor.execute("SELECT sql FROM sqlite_master WHERE type='table' AND name='your_table_name';")
table_info = cursor.fetchone()
if table_info:
create_statement = table_info[0]
column_names = create_statement.split('(')[1].split(')')[0].split(',')
column_names = [col.strip().split(' ')[0] for col in column_names]
print("Column Names:", column_names)
connection.close()
Output:
Column Names: ['id', 'name', 'age', 'email']
In this example, we first connect to the SQLite database and create a cursor. We then execute a SQL query on the sqlite_master
table to retrieve the CREATE TABLE
statement for the specified table. The fetchone()
method gets the first row of the result, which contains the SQL statement. We then parse this statement to extract the column definitions. By splitting the string appropriately, we isolate the column names and clean them up. This method is particularly useful when you need to understand the structure of a table without relying on PRAGMA
.
Method 3: Using the SQLite Command Line Interface
If you’re working directly with SQLite via the command line, you can easily retrieve column names using a simple SQL command. This method is user-friendly and provides immediate results. Here’s how you can do it.
.headers on
.mode column
PRAGMA table_info(your_table_name);
Output:
id INTEGER
name TEXT
age INTEGER
email TEXT
In the command line interface, you first enable headers and set the output mode to column for better readability. Then, you execute the PRAGMA table_info(your_table_name)
command, which displays the column names along with their data types in a structured format. This method is ideal for quick checks and provides a clear view of the table structure directly in the terminal.
Conclusion
Retrieving column names from an SQLite database is a fundamental skill for anyone working with databases. Whether you choose to use the PRAGMA
statement, query the sqlite_master
table, or utilize the command line interface, each method has its advantages. By mastering these techniques, you can efficiently manage your SQLite databases and enhance your data handling capabilities. Remember, understanding the structure of your tables is key to effective database management. Happy coding!
FAQ
-
How can I get column names in SQLite without using Python?
You can use thePRAGMA table_info(your_table_name);
command in the SQLite command line interface to retrieve column names directly. -
Is it possible to get column names for all tables in an SQLite database?
Yes, you can query thesqlite_master
table to get a list of all tables, and then usePRAGMA table_info(table_name);
for each table to retrieve their column names.
-
Can I retrieve column names using a graphical SQLite client?
Yes, most graphical SQLite clients provide a way to view table structures, including column names, through their user interface. -
What is the difference between
PRAGMA
and queryingsqlite_master
?
PRAGMA table_info()
provides detailed information about the columns of a specific table, while queryingsqlite_master
gives you the SQL statement used to create the table, which you can parse to get column names. -
Are there any performance considerations when retrieving column names?
Generally, retrieving column names is a lightweight operation, but if you are working with a large number of tables, queryingsqlite_master
might be more efficient than running multiplePRAGMA
commands.