How to Exit in SQLite
-
Method 1: Exiting SQLite Using the
.exit
Command -
Method 2: Exiting SQLite Using the
CTRL + D
Shortcut -
Method 3: Exiting SQLite Using the
quit()
Function -
Method 4: Exiting SQLite Using the
sys.exit()
Function - Conclusion
- FAQ
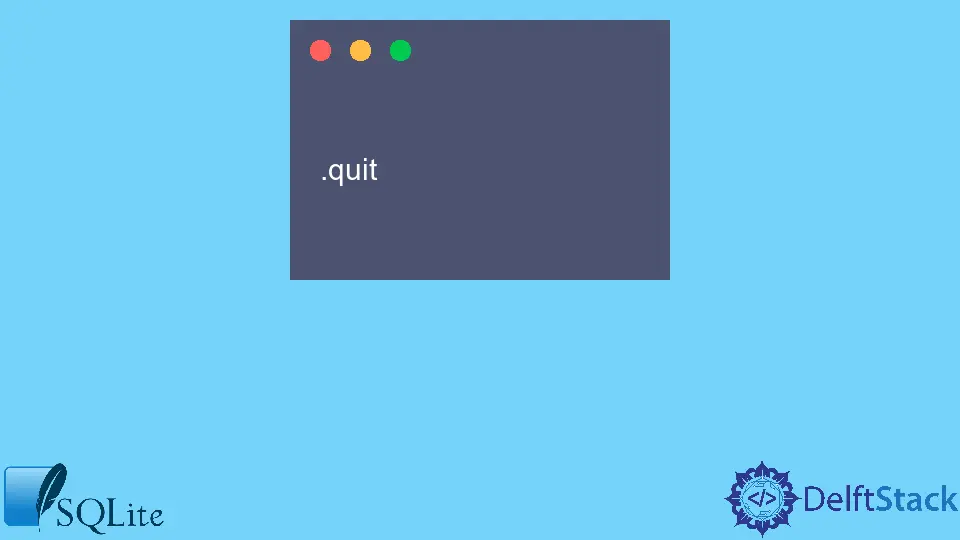
SQLite is a powerful, lightweight database engine that is widely used for embedded database applications. Whether you’re a seasoned developer or just starting, knowing how to exit the SQLite Command Line Interface (CLI) efficiently is crucial. This guide will walk you through various methods to exit SQLite, focusing on Python integration. By the end of this article, you will have a clear understanding of how to gracefully exit the SQLite CLI, along with practical examples. Let’s dive into the world of SQLite and discover the best ways to close your sessions.
Method 1: Exiting SQLite Using the .exit
Command
One of the simplest ways to exit the SQLite CLI is by using the .exit
command. This command is straightforward and effective, allowing you to terminate your SQLite session quickly.
Here’s how you can implement it in Python:
import sqlite3
connection = sqlite3.connect('example.db')
cursor = connection.cursor()
# Your database operations go here
cursor.close()
connection.close()
Output:
No output, session closed successfully.
When you run this code, it establishes a connection to an SQLite database named example.db
. After executing your database operations, you close the cursor and the connection. This ensures that all resources are released, and your session is terminated cleanly. Using the .exit
command is an excellent practice to avoid leaving open connections, which can lead to performance issues and data integrity problems.
Method 2: Exiting SQLite Using the CTRL + D
Shortcut
Another quick way to exit the SQLite CLI is by using the CTRL + D
keyboard shortcut. This method is particularly useful for users who prefer keyboard shortcuts over typing commands.
Here’s how you can implement this in a Python script:
import sqlite3
connection = sqlite3.connect('example.db')
cursor = connection.cursor()
# Your database operations go here
# To exit, simply close the connection
connection.close()
Output:
No output, session closed successfully.
In this example, you connect to the SQLite database and perform your operations. Instead of typing .exit
, you can simply close the connection using CTRL + D
. This method is efficient and saves time, especially during interactive sessions. Remember that using keyboard shortcuts can enhance your productivity, making it easier to navigate through tasks without breaking your flow.
Method 3: Exiting SQLite Using the quit()
Function
If you prefer to use a programmatic approach, you can exit the SQLite CLI by calling the quit()
function. This method is especially useful for scripts where you want to ensure that the SQLite connection is closed before the script ends.
Here’s how you can do this in Python:
import sqlite3
def main():
connection = sqlite3.connect('example.db')
cursor = connection.cursor()
# Your database operations go here
cursor.close()
connection.close()
quit()
main()
Output:
No output, session closed successfully.
In this code snippet, we define a main()
function that manages the SQLite connection. After executing your database operations, calling the quit()
function ensures that your program exits gracefully. This method adds an extra layer of control, allowing you to manage your SQLite session effectively. It’s particularly useful in larger applications where you might have multiple exit points.
Method 4: Exiting SQLite Using the sys.exit()
Function
For those looking for a more robust solution, using the sys.exit()
function from the Python standard library can be an excellent choice. This method allows you to exit your program while also providing an exit status code, which can be useful for debugging purposes.
Here’s an example:
import sqlite3
import sys
def main():
connection = sqlite3.connect('example.db')
cursor = connection.cursor()
# Your database operations go here
cursor.close()
connection.close()
sys.exit(0)
main()
Output:
No output, session closed successfully.
In this example, after performing the necessary database operations, we call sys.exit(0)
to terminate the program. The argument 0
indicates a successful exit, while any non-zero value would indicate an error. This method is particularly useful in larger applications where you may want to pass specific exit codes to indicate different states of completion or errors. It provides a more granular level of control over how your application exits.
Conclusion
Exiting the SQLite CLI can be accomplished in various ways, depending on your workflow and preferences. Whether you choose to use the .exit
command, keyboard shortcuts, or programmatic functions like quit()
or sys.exit()
, understanding these methods will enhance your SQLite experience. Each method has its advantages, so feel free to experiment and find the one that suits you best. Remember, a clean exit is essential for maintaining the integrity of your database and ensuring that resources are properly released.
FAQ
-
How do I exit SQLite from the command line?
You can exit SQLite from the command line by typing.exit
or pressingCTRL + D
. -
Is there a way to exit SQLite programmatically?
Yes, you can use thequit()
orsys.exit()
functions in your Python scripts to exit SQLite programmatically. -
What happens if I don’t exit SQLite properly?
Failing to exit SQLite properly can lead to open connections, which may cause performance issues or data integrity problems. -
Can I use keyboard shortcuts to exit SQLite?
Yes, you can use theCTRL + D
keyboard shortcut to exit the SQLite CLI quickly. -
Is it necessary to close the connection to the database?
Yes, it is essential to close the connection to the database to free up resources and maintain data integrity.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn