How to Create Table if Not Exists in SQLite
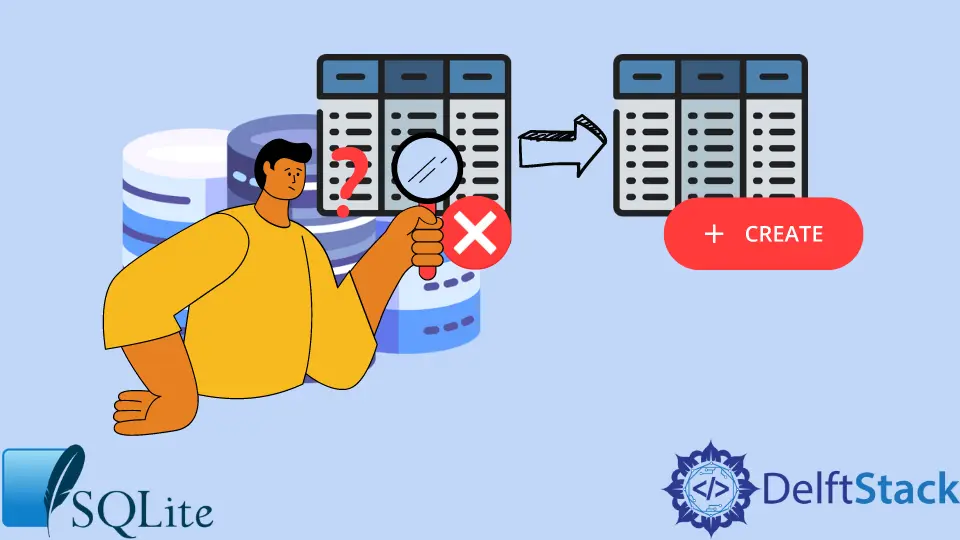
When you are working with a large database, there might be a large number of interconnected tables. So when you try to include one more table in the database, you must select a new name for the table.
But there’s an easy way to check if the selected name for the new table already exists so that it won’t conflict with the other table.
This article shows us how to check whether the selected name for the new table already exists. Also, we will see an example to make the topic easier.
We are going to use SQLite in this article. We will use some built-in keywords in SQLite for this purpose: IF NOT EXISTS
.
Use CREATE TABLE IF NOT EXISTS
in SQLite
In our below example, we will see how we can check the existence of a table. The SQL query we will use is below.
CREATE TABLE IF NOT EXISTS New_table (
id INTEGER,
Name VARCHAR(25)
);
In the query above, we will check whether the New_table
already exists by using the keywords IF NOT EXISTS
. If it doesn’t exist, we will create one using the keyword CREATE TABLE
.
When you execute the query above, you will have a new table in your database. But if the table New_table
already exists, then the database remains unchanged.
The method we used in this article is safe to use when the database is very large, all the tables are interconnected, and one unexpected change in the database causes a serious effect on the whole database structure.
Please note that the queries we provided in this article are written for SQLite.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn