How to Convert String Date With Format in SQLite
- Understanding Date Formats in SQLite
- Converting String Dates Using SQLite Functions
- Handling Different Date Formats
- Conclusion
- FAQ
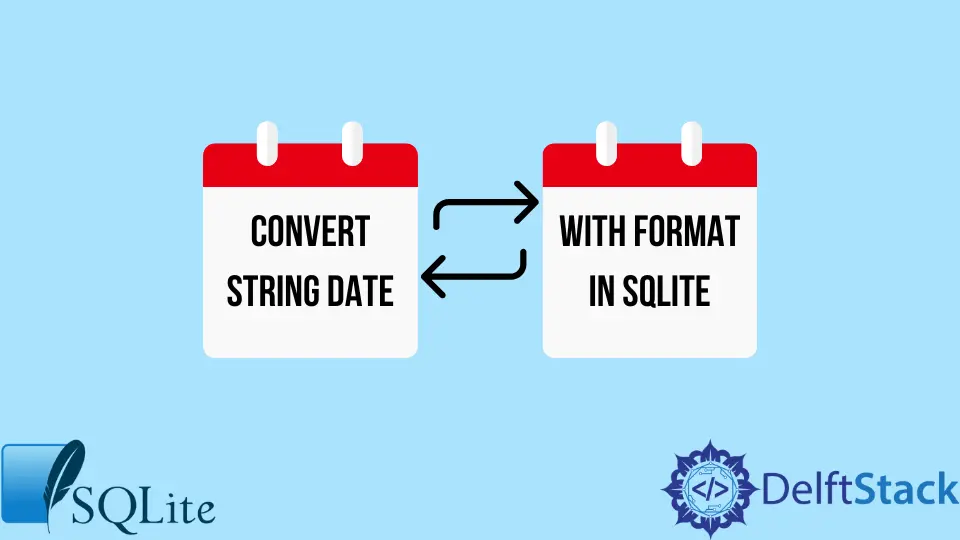
When working with databases, handling dates and times can often be a bit tricky, especially when those dates come in string format. SQLite, a lightweight database engine, provides various functions to manipulate and convert date strings into a format that can be easily used in queries.
In this tutorial, we will explore how to convert string dates with specific formats in SQLite. By the end of this article, you will be equipped with the knowledge to effectively manage date strings in your SQLite databases, ensuring your data is both accurate and easy to work with.
Understanding Date Formats in SQLite
SQLite supports several date and time formats, including ISO8601, Julian day numbers, and Unix timestamps. However, when your date is stored as a string, it may not always conform to these formats. This can lead to challenges when performing date calculations or comparisons. The key to converting string dates in SQLite is understanding the format of the string you are working with.
For instance, if your date is in the format “MM-DD-YYYY,” you will need to convert it to a format that SQLite recognizes. SQLite’s built-in date and time functions, such as date()
, time()
, and datetime()
, can help with this conversion.
Converting String Dates Using SQLite Functions
SQLite provides several functions that can be utilized to convert string dates into a recognizable format. Here, we will explore the strftime()
function, which is particularly useful for formatting dates.
Using strftime() for Date Conversion
The strftime()
function formats a date string based on the specified format. Here’s how you can use it:
SELECT strftime('%Y-%m-%d', '12-31-2022');
Output:
2022-12-31
In this example, we are converting the date string ‘12-31-2022’ into the format ‘YYYY-MM-DD’. The %Y
, %m
, and %d
format specifiers represent the year, month, and day, respectively. By specifying the desired format, you can transform any string date into a format that SQLite recognizes.
To further illustrate, let’s say you have a table named events
with a column event_date
containing dates in the ‘MM-DD-YYYY’ format. You can convert and select these dates using:
SELECT strftime('%Y-%m-%d', event_date) AS formatted_date FROM events;
Output:
2023-01-01
2023-02-15
This command will convert all the dates in the event_date
column to the ‘YYYY-MM-DD’ format, making it easier to perform date comparisons or calculations.
Handling Different Date Formats
Sometimes, your string dates may come in various formats. SQLite allows you to handle these variations through conditional logic or by chaining multiple functions.
Converting Multiple Formats
If you have dates in different formats, you can use a combination of CASE
statements and strftime()
to handle the conversions. Here’s an example:
SELECT
CASE
WHEN event_date LIKE '%-%' THEN strftime('%Y-%m-%d', event_date)
WHEN event_date LIKE '%/%' THEN strftime('%Y-%m-%d', replace(event_date, '/', '-'))
ELSE event_date
END AS formatted_date
FROM events;
Output:
2022-12-31
2022-01-15
In this example, we check if the date contains a hyphen or a slash. If it contains a slash, we replace it with a hyphen and then format it. This method ensures that various string date formats can be converted seamlessly.
Conclusion
Converting string dates in SQLite is a crucial skill for anyone working with databases. By leveraging SQLite’s built-in functions like strftime()
, you can transform string dates into a format that is both recognized and usable within your queries. Whether you are dealing with a single date format or multiple formats, the methods outlined in this tutorial will help ensure your date handling is accurate and efficient.
With these techniques, you can confidently manage and manipulate date strings in your SQLite databases, paving the way for more effective data analysis and reporting.
FAQ
-
How do I convert a string date to a date format in SQLite?
You can use thestrftime()
function to convert a string date into a recognized date format. -
What formats does SQLite support for dates?
SQLite supports formats like ISO8601, Julian day numbers, and Unix timestamps.
-
Can I convert multiple date formats in SQLite?
Yes, you can useCASE
statements along withstrftime()
to handle multiple date formats. -
What happens if my date string is not in a recognized format?
If your date string is not in a recognized format, SQLite may return NULL or an error when you attempt to convert it. -
Is it possible to format dates in SQLite for display purposes?
Absolutely! Thestrftime()
function can be used to format dates for display in various styles.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn