How to Find Data That Contains Matching Pattern in SQLite
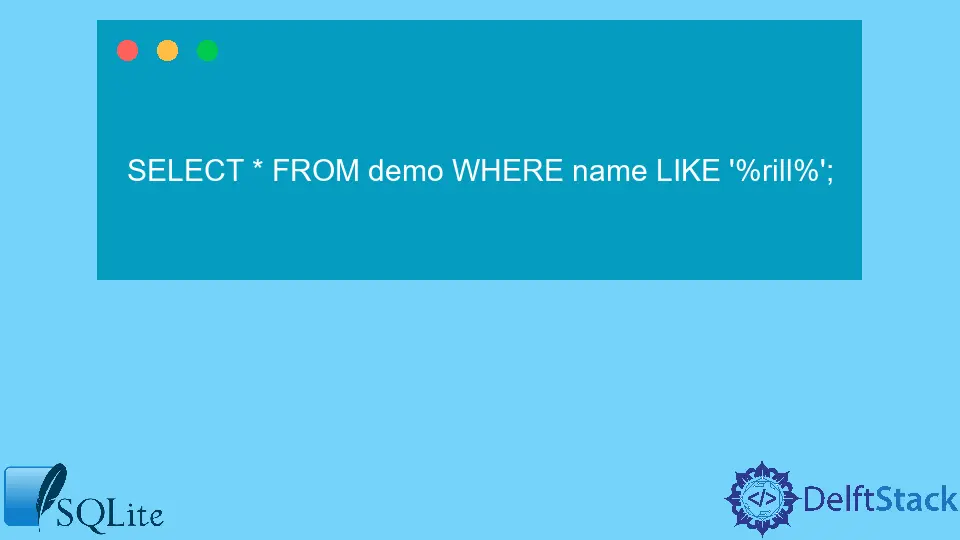
Sometimes we need to search for specific data from a database that matches the criteria. For example, we may need to find specific data that contains New
in front of the city name.
In this article, we will see how to find specific data from databases that match the specific criteria in SQLite and an example related to the topic with a proper explanation to make the topic easier.
Find Specific Data Based on Matching Criteria
In our below example query, we will search for a name
that contains rill
in the demo
table. Have a look at the below example.
SELECT * FROM demo WHERE name LIKE '%rill%';
In the above query, we have shown all the data from the table demo
where the name
contains the pattern rill
. Here the keyword LIKE
use to match a specific pattern, and the symbol *
means all the fields related to the data.
When you run the above example query, you will get this output.
We need this method when we don’t know the unique key like id
but know a specific data pattern.
Please note that the query we provided in this article is written for SQLite.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn