How to Check if Table Exists in SQLite Database
- Method 1: Using the SQLite PRAGMA Statement
- Method 2: Using a Try-Except Block
- Method 3: Querying the Information Schema
- Conclusion
- FAQ
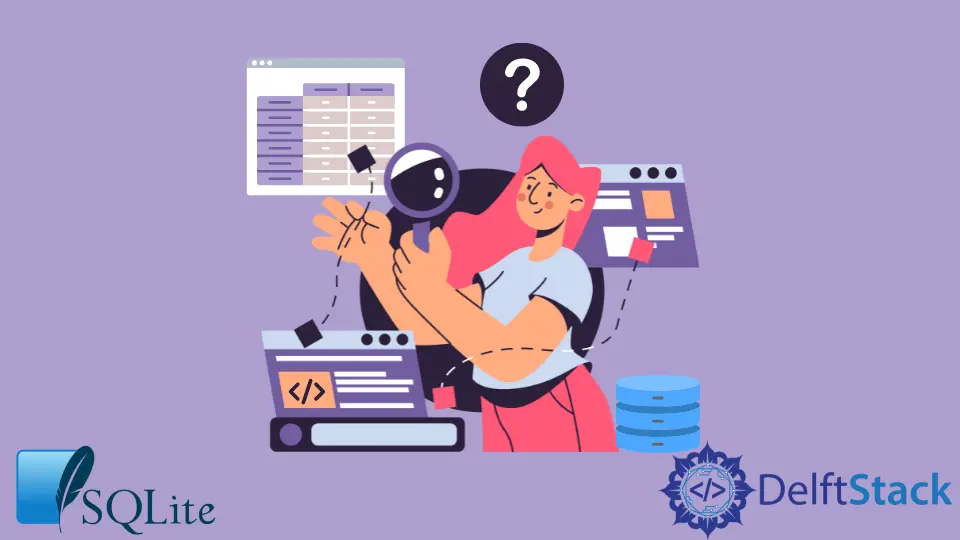
When working with databases, one common task is verifying the existence of a table before performing operations on it. This is especially crucial in SQLite, a lightweight database engine widely used in applications. Knowing how to check if a table exists can save you from unnecessary errors and streamline your database management.
In this article, we will explore different methods to check for a table’s existence in an SQLite database using Python. Whether you’re a beginner or an experienced developer, these techniques will enhance your programming toolkit. Let’s dive in!
Method 1: Using the SQLite PRAGMA Statement
One of the simplest ways to check if a table exists in an SQLite database is by using the PRAGMA statement. This method involves querying the sqlite_master
table, which stores information about all the tables, indexes, and views in your database. By executing a simple SQL query, you can determine if your desired table is present.
Here’s how you can do it in Python:
import sqlite3
def table_exists(db_name, table_name):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute(f"SELECT name FROM sqlite_master WHERE type='table' AND name='{table_name}';")
result = cursor.fetchone()
conn.close()
return result is not None
# Example usage
db_name = 'example.db'
table_name = 'users'
exists = table_exists(db_name, table_name)
print(exists)
Output:
True or False
In this code, we first establish a connection to the SQLite database using sqlite3.connect()
. We then create a cursor object to execute SQL commands. The query checks the sqlite_master
table for a record matching the specified table name. If a match is found, fetchone()
returns the name of the table; otherwise, it returns None
. Finally, we close the connection and return a boolean indicating whether the table exists.
Method 2: Using a Try-Except Block
Another effective way to check if a table exists in an SQLite database is by attempting to select data from the table within a try-except block. This method is straightforward and can be particularly useful if you plan to access the table immediately after checking for its existence.
Here’s a Python code snippet demonstrating this approach:
import sqlite3
def check_table_exists(db_name, table_name):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
try:
cursor.execute(f"SELECT * FROM {table_name} LIMIT 1;")
return True
except sqlite3.Error:
return False
finally:
conn.close()
# Example usage
db_name = 'example.db'
table_name = 'orders'
exists = check_table_exists(db_name, table_name)
print(exists)
Output:
True or False
In this example, we connect to the SQLite database and create a cursor. The code attempts to execute a simple SELECT
query on the specified table. If the table does not exist, an sqlite3.Error
is raised, and we catch this exception to return False
. If the query executes successfully, we return True
. The finally
block ensures that the database connection is closed regardless of whether an error occurred.
Method 3: Querying the Information Schema
SQLite doesn’t have a traditional information schema like other database systems, but you can still query the sqlite_master
table to gather information about your tables. This method is similar to the first one but allows for more complex queries if needed.
Here’s how you can implement this in Python:
import sqlite3
def query_table_info(db_name, table_name):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute("SELECT name FROM sqlite_master WHERE type='table';")
tables = cursor.fetchall()
conn.close()
return table_name in [table[0] for table in tables]
# Example usage
db_name = 'example.db'
table_name = 'products'
exists = query_table_info(db_name, table_name)
print(exists)
Output:
True or False
In this code, we connect to the database and execute a query that retrieves all table names from the sqlite_master
table. The result is fetched and stored in a list. We then check if the specified table name is present in this list. This method is particularly useful if you want to list all tables or perform additional checks.
Conclusion
Checking if a table exists in an SQLite database is a fundamental task that can prevent errors and improve your application’s robustness. In this article, we explored three effective methods using Python: querying the sqlite_master
table with PRAGMA, using a try-except block, and querying the information schema. Each method has its advantages, so you can choose the one that best fits your needs. With these techniques in your toolkit, you’ll be well-equipped to manage your SQLite databases efficiently.
FAQ
-
how can I check if a table exists in SQLite without using Python?
You can use the SQLite command line tool and execute a query on the sqlite_master table to check for the table’s existence. -
what happens if I try to access a non-existing table in SQLite?
An error will be raised, specifically an sqlite3.Error, indicating that the table does not exist.
-
can I check for the existence of a view in SQLite using the same methods?
Yes, you can modify the queries to check for views by changing the type in the sqlite_master table from ’table’ to ‘view’. -
is there a performance difference between the methods discussed?
The performance difference is negligible for small databases, but using a try-except block may be slightly faster when you immediately need to access the table. -
can I use these methods in a multi-threaded application?
Yes, SQLite is thread-safe, and these methods can be used in multi-threaded applications, but ensure proper connection management.