How to Open .Sqlite File in Windows
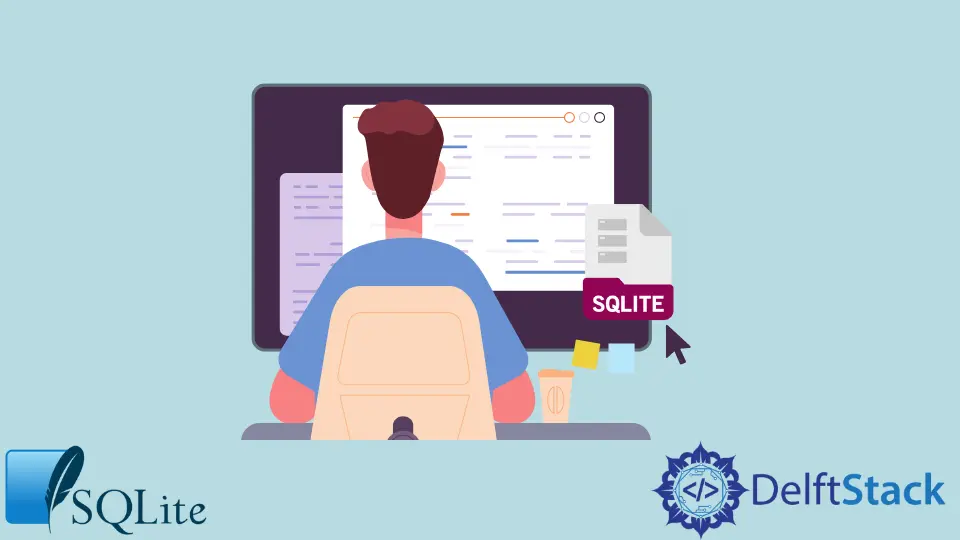
SQLite supports two kinds of extensions to save the database: .sqlite
and .db
. But both are the same.
If you are using the Command Line Interface for SQLite, it’s easy to open SQLite files.
This article will show how we can open a .sqlite
file using Windows Command Prompt. Let’s look at the steps to open a .sqlite
file in Windows.
Open the .sqlite
File
To open the .sqlite
file, you need to execute the command below.
sqlite3 mydata.sqlite
Check Whether the File Is Successfully Opened
To check whether the file was successfully opened or not. You can execute the command .tables
, showing you the list of tables inside the database.
After doing all the steps, you will get the below output.
Please note that the file types
.sqlite
and.db
are the same.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn