The yield Keyword in Scala Programming Language
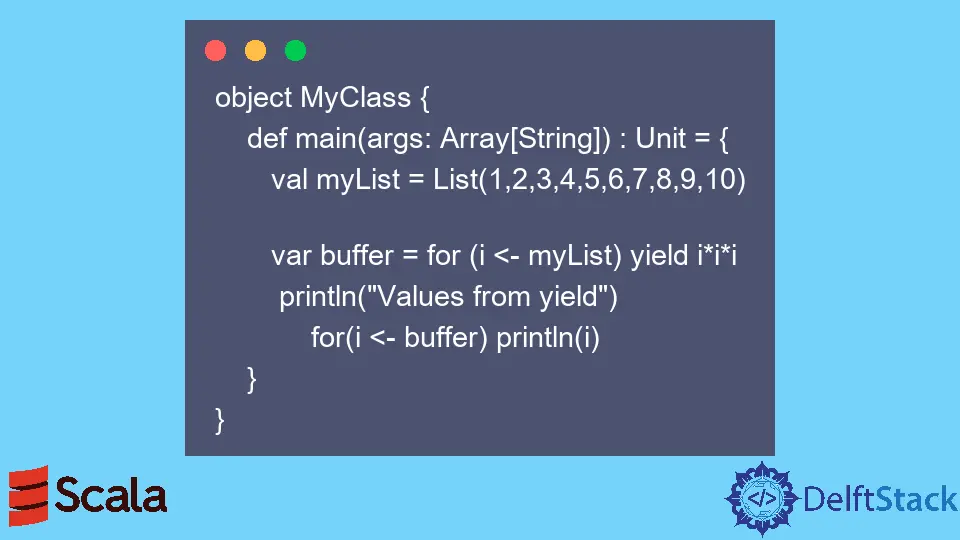
In this article, we will learn how to use the yield
keyword in Scala with the help of different examples.
The yield
keyword is used with for
loops. In each iteration of the for
loop, yield
generates a remembered value.
It acts as a buffer for the for
loop. For each iteration of the for
loop, items are added to this buffer.
Once the for
loop finishes all its iterations, a collection of all the yielded values is returned.
The type of collection that is returned. The same type for
loop was iterating, so a List
yields a List
, a Map
yields a Map
, an array
yields an array
, and so on.
The syntax of yield
:
for() yield variable;
Using the yield
Keyword in an Array in Scala
We will iterator over all the elements of an array using for loop and use the yield
keyword to store elements at each iteration of the for
loop.
Example code:
object MyClass {
def main(args: Array[String]) : Unit = {
val array = Array(100,200,300,500,600)
var buffer = for (i <- array if i > 200) yield i
for(i<-buffer)
{
println(i)
}
}
}
Output:
300
500
600
yield
With Scala List
We will iterate over all the elements of a List
using for loop and use the yield
keyword to store elements at each iteration of the for
loop and perform some operation on them.
object MyClass {
def main(args: Array[String]) : Unit = {
val myList = List(1,2,3,4,5,6,7,8,9,10)
var buffer = for (i <- myList) yield i*i*i
println("Values from yield")
for(i <- buffer) println(i)
}
}
Output:
Values from yield
1
8
27
64
125
216
343
512
729
1000