The Underscore Placeholder in Scala
- What is the Underscore Placeholder?
- Using Underscore in Anonymous Functions
- Underscore in Pattern Matching
- Underscore in Partially Applied Functions
- Conclusion
- FAQ
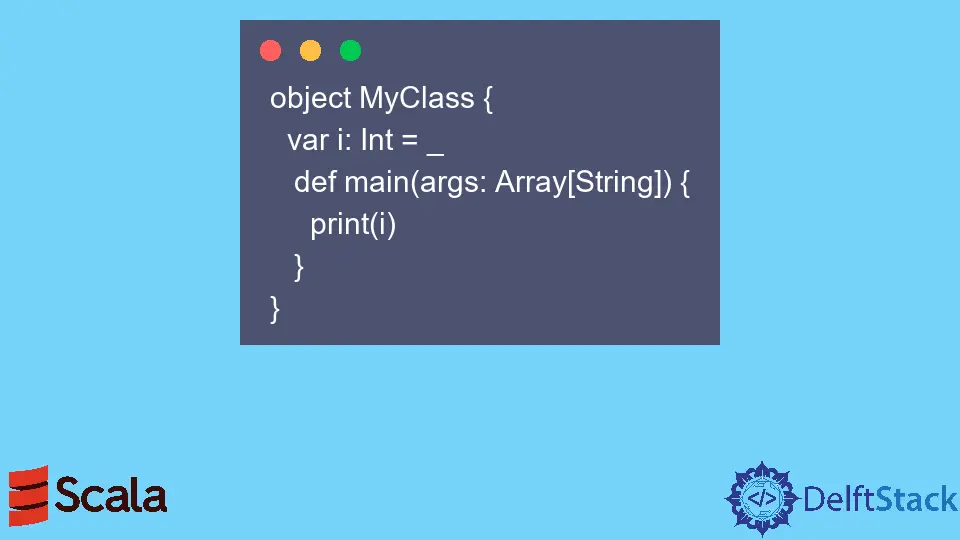
In the world of Scala, the underscore placeholder is a powerful feature that often goes unnoticed by many developers. This seemingly simple symbol can dramatically enhance the way we write code, making it more concise and expressive. Whether you’re a seasoned Scala programmer or just starting your journey, understanding how to use the underscore placeholder can streamline your code and improve readability.
In this article, we will explore what the underscore placeholder is, its various uses, and how it can simplify your Scala programming tasks. By the end, you’ll see how this little symbol can pack a big punch in your code.
What is the Underscore Placeholder?
The underscore placeholder in Scala serves multiple purposes. At its core, it acts as a shorthand for anonymous functions, making your code cleaner and easier to read. Instead of defining a full function, you can use the underscore to represent parameters in a more succinct way. This feature is especially useful in functional programming, where functions are first-class citizens.
For example, consider a scenario where you want to apply a function to a collection of numbers. Instead of writing out a complete function definition, you can simply use the underscore to indicate the parameter. This not only saves time but also reduces boilerplate code.
Here’s a basic example:
val numbers = List(1, 2, 3, 4, 5)
val squares = numbers.map(x => x * x)
This can be simplified using the underscore placeholder:
val squares = numbers.map(x => x * x)
Output:
List(1, 4, 9, 16, 25)
In this example, x
is replaced by _
, making the code cleaner and more readable.
Using Underscore in Anonymous Functions
One of the most common uses of the underscore placeholder is in anonymous functions. In Scala, you can define functions without explicitly naming them, which is particularly useful for short operations. The underscore acts as a placeholder for parameters, allowing you to write concise, expressive code.
Consider the following example where we want to filter a list of numbers to find even numbers. Instead of writing a full function, we can leverage the underscore for brevity.
val numbers = List(1, 2, 3, 4, 5, 6)
val evens = numbers.filter(_ % 2 == 0)
Output:
List(2, 4, 6)
In this code snippet, the underscore replaces the parameter in the filter function, making it clear and easy to read. The use of the underscore here eliminates the need for verbose syntax, allowing developers to focus on the logic rather than the boilerplate.
This practice not only enhances readability but also encourages a functional programming style that is more aligned with Scala’s design principles.
Underscore in Pattern Matching
Another fascinating use of the underscore placeholder is in pattern matching. In Scala, pattern matching is a powerful feature that allows you to deconstruct data structures easily. The underscore can be used as a wildcard, representing any value. This is particularly useful when you want to ignore certain values in a pattern match.
Here’s an example of using the underscore in pattern matching:
val tuple = (1, "Scala", true)
tuple match {
case (x, _, z) => println(s"x: $x, z: $z")
}
Output:
x: 1, z: true
In this example, the underscore is used to ignore the second element of the tuple. This allows us to focus on the values we care about without cluttering our code with unnecessary variables. The underscore acts as a placeholder for any value, making pattern matching more flexible and expressive.
Understanding how to use the underscore in pattern matching can significantly improve the clarity of your code, especially when dealing with complex data structures.
Underscore in Partially Applied Functions
The underscore placeholder is also instrumental in creating partially applied functions. A partially applied function is a function that is not fully applied to its arguments. This allows you to create new functions by fixing some of the parameters of an existing function.
Consider the following example where we have a function that takes two parameters:
def add(a: Int, b: Int): Int = a + b
We can create a partially applied function using the underscore:
val addFive = add(5, _: Int)
Output:
addFive(10) // returns 15
In this case, addFive
is a new function that adds 5 to any integer passed to it. The underscore acts as a placeholder for the second parameter, allowing us to create a new function with just one argument.
This technique is particularly useful in functional programming, where functions are often treated as first-class citizens. By leveraging the underscore, you can create more modular and reusable code.
Conclusion
The underscore placeholder in Scala is a versatile tool that can greatly enhance your coding experience. Whether you’re simplifying anonymous functions, streamlining pattern matching, or creating partially applied functions, the underscore can help you write cleaner, more expressive code. As you continue to explore Scala, keep an eye out for opportunities to use this powerful feature. By mastering the underscore placeholder, you’ll be well on your way to becoming a more efficient and effective Scala programmer.
FAQ
-
What is the purpose of the underscore placeholder in Scala?
The underscore placeholder is used to simplify code by acting as a shorthand for anonymous functions, wildcards in pattern matching, and placeholders in partially applied functions. -
How do I use the underscore in anonymous functions?
You can use the underscore to represent parameters in anonymous functions, making your code more concise and readable. -
Can the underscore be used in pattern matching?
Yes, the underscore can be used as a wildcard in pattern matching to ignore certain values while focusing on the ones you care about. -
What are partially applied functions, and how does the underscore help?
Partially applied functions are functions that are not fully applied to their arguments. The underscore acts as a placeholder for the missing parameters, allowing you to create new functions with fewer arguments.
- Why should I use the underscore placeholder in my Scala code?
Using the underscore placeholder can make your code cleaner, more expressive, and easier to read, aligning with Scala’s functional programming principles.