try...catch in Scala
-
Scala Code Without a
Try-Catch
Block -
Use the
Try-Catch
Block to Enclose the Suspect Code and to Handle the Exception in Scala -
Use the
Try-Catch
andFinally
Block to Execute Code Inside the Body in Scala
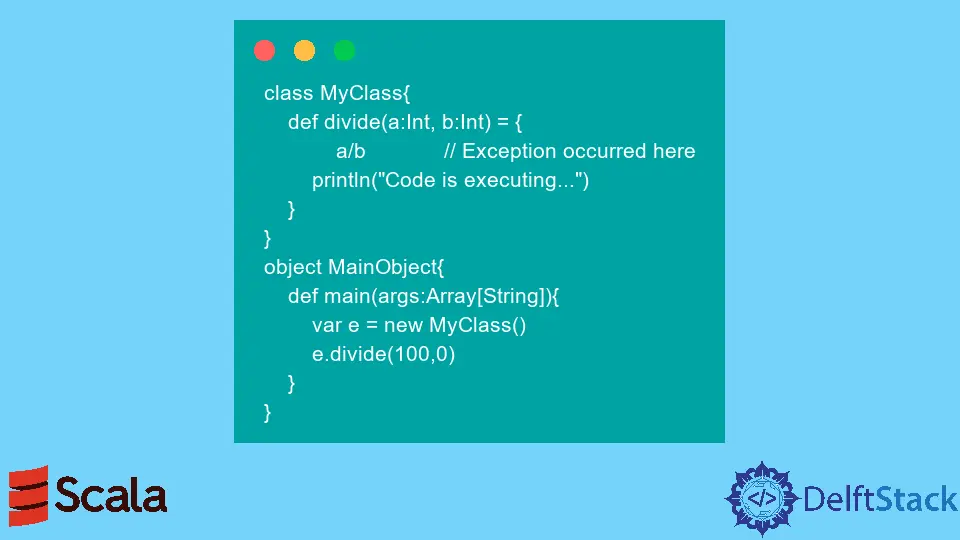
The try-catch
block is the essential handler block in Scala that is used for exception
handling.
An exception
is an abnormal condition or code flow that leads to codetermination and stops with unexpected output.
The try
block is used to enclose the suspect code, and the catch
is used to handle the exception and provide the necessary details to debug.
Scala Code Without a Try-Catch
Block
In this scenario, we don’t use the try-catch
blocks and see what happens if our code fails to execute.
class MyClass{
def divide(a:Int, b:Int) = {
a/b // Exception occurred here
println("Code is executing...")
}
}
object MainObject{
def main(args:Array[String]){
var e = new MyClass()
e.divide(100,0)
}
}
Output:
java.lang.ArithmeticException: / by zero
at MyClass.divide(jdoodle.scala:4)
Here, a user provides a wrong input and dividing by zero compiler stops the execution unexpectedly.
Use the Try-Catch
Block to Enclose the Suspect Code and to Handle the Exception in Scala
Here, we enclosed the suspect code into the try
block and provided the catch
handler’s necessary information.
If any exception occurs, then the code of the catch
handler will execute only. Otherwise, the code will normally execute with the desired result.
class MyClass{
def divide(a:Int, b:Int) = {
try{
a/b
}catch{
case e: ArithmeticException => println(e)
}
println("Code is executing...")
}
}
object MainObject{
def main(args:Array[String]){
var e = new MyClass()
e.divide(10,0)
}
}
Output:
java.lang.ArithmeticException: / by zero
Code is executing...
Use the Try-Catch
and Finally
Block to Execute Code Inside the Body in Scala
The finally
block executes the code inside its body no matter what exception occurs in the code.
It guarantees to execute code in all circumstances except for disastrous change.
class MyClass{
def divide(a:Int, b:Int) = {
try{
a/b
}catch{
case e: ArithmeticException => println(e)
}finally{
println("Finaly block always executes")
}
println("Code is executing...")
}
}
object MainObject{
def main(args:Array[String]){
var e = new MyClass()
e.divide(10,0)
}
}
Output:
java.lang.ArithmeticException: / by zero
Finaly block always executes
Code is executing...